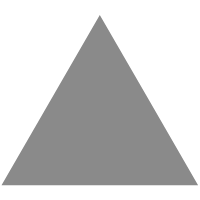
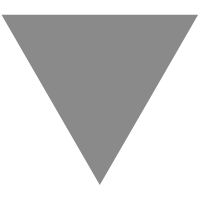
Machine Learning using TensorFlow and PyCharm Series: Part 1: Setting up environ...
source link: https://www.tuicool.com/articles/hit/63MR3mN
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
TensorFlow is an open source computation framework for building machine learning models. Its design make use of lessons learnt from earlier machine learning frameworks — Torch, Theano, Caffe, and Keras. Torch is the earliest machine learning framework that made of the term Tensor. Theano makes use of Graph data structure to store operations and compile them to high-performance code. Caffe is a high performance framework written in C++ and makes feasible to execute applications on different devices. Keras provides an easy to use API to interface with various machine learning frameworks like Theano.
TensorFlow makes use of all the good design decisions made by the earlier four framework TensorFlow name is made up of two words – Tensor and Flow. Tensor means a multi-dimensional array. Flow means a graph of operations. It’s core is written in C++ and CUDA(Nvidia’s language for programming GPUs). It provides a high level API in Python, C, Java, Go,Rust, JavaScript and Swift that programmers can use to build their applications.
TensorFlow is composed of two parts:
- A library to define computational graphs
- A runtime to execute graphs on a variety of different hardware. It supports execution on both CPUs and GPUs.
Installing TensorFlow on your machine with PyCharm
I am going to use PyCharm IDE by JetBrains for working with TensorFlow. PyCharm is a Python IDE that provides code completion, refactoring , and in-built support for running Jupyter notebooks.
Start by downloading Python 3 from the official website .
After installing Python, download and install PyCharm from its official website . We are going to use Community edition of PyCharm.
Open the PyCharm application and you will see following screen.
Click on Create New Project
and specify location and select New environment using Virtualenv
. Virtualenv avoids polluting the global python installation. We can work with different versions of python for different projects.
Press Create
after entering the details. It will create the project and open a new window as shown below.
Next, we will install TensorFlow library using PyCharm itself. On Mac, go to PyCharm preferences as shown below.
Once Preferences window is open, go to Project > Project Interpreter as shown below.
Click on the +
icon in left bottom and you will get a window in which you can search TensorFlow module as shown below.
Click on Install Package
to install TensorFlow. It might take a couple of minutes depending on your internet speed. Once installed you will get Packages installed successfully Installed packages: 'tensorflow'.
Working with TensorFlow API
Create a directory named src
inside the 01-tensorflow-intro
directory. We will use it to store Python files.
Create a ne Python file 01.py
inside the src
directory. We will write our first TensorFlow program inside it.
We will start by creating a simple TensorFlow program to add two numbers.
import tensorflow as tf # 1 a = tf.constant(1) # 2 b = tf.constant(2) # 3 c = tf.add(a, b) # 4 with tf.Session() as sess: # 5 print(sess.run(c)) # 6
The code shown above does the following:
- It imports the
tensorflow
module and give it an aliastf
- We created two constant tensors named a and b. As mentioned before, tensor is a multidimensional array. What might confuse you is that
a
andb
does not refer to arrays. They are constant values represented as unit arrays. If you printa
, the output will be followingTensor("Const:0", shape=(), dtype=int32)
.Const:0
is the autogenerated name of the Tensor.()
is the shape of the tensor. Andint32
is the datatype. - Next, we defined another Tensor
c
uses thesum
operator to add botha
andb
tensors. - The line 5 creates as instance of
Session
class and names itsess
. Session provides an environment in which TensorFlow operations are executed. - Using the session created in the line 5, we run the tensor
c
that performed addition ofa
andb
tensors.
To run the code example above, you can right click anywhere on the file and run the program as shown in the image below.
You will see the output 3
in the console.
Key points about TensorFlow API
The two key points that you should be aware of TensorFlow API are:
- TensorFlow API is by default lazy. It does not perform any computation till you call
sess.run()
. If you printc
after line 4 you will get following outputTensor("Add:0", shape=(), dtype=int32)
. As you can see, we didn’t see the result3
in the print statement. Nothing will get executed. We have only defined our computation graph. This is the difference between declarative programming and imperative programming. - TensorFlow will only execute necessary portion of the graph. In the example above, it will evaluate everything but it different example it might execute a sub-portion of the graph.
Conclusion
In the first part of this series, we have set up our TensorFlow environment and written our first TensorFlow program. In the next part, we will get deeper into TensorFlow API covering Tensors and Graphs in detail.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK