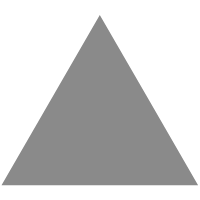
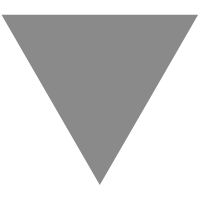
C# 7.2 – Let’s talk about readonly structs
source link: https://www.tuicool.com/articles/hit/bQVNJj6
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
With the Roslyn update, this was the first time that C# received minor versions instead of only major ones. Roslyn helped the designers of the language to move in different leaps and provide more stuff in shorter time with minor versions.
In this article we will be discussing about a feature introduced in C# 7.2, the readonly structs
Any struct can have public properties, private properties accessors etc. For our examples we will start with the following struct.
public struct Person { public string Name { get; set; } public string Surname { get; set; } public int Age { get; set; } public Person(string name, string surname, int age) { Name = name; Surname = surname; Age = age; } public void Replace(Person other) { this = other; } }
As you can see, all properties can be publicly accessible and edited. Heck, we even have access to the content of this
and change it to another instance of the same struct.
Well this is where the readonly
keyword comes in place. If add it in the struct definition like the following
public readonly struct Person { public string Name { get; set; } public string Surname { get; set; } public int Age { get; set; } public Person(string name, string surname, int age) { Name = name; Surname = surname; Age = age; } public void Replace(Person other) { this = other; } }
The following screenshot shows the result of compiling the above code
So what happened? Well by adding the readonly
keyword to our struct we have basically made every single property readonly, including the value of this
.
The only way to make our code compile is to make everything readonly, which means our struct would look like this
public readonly struct Person { public string Name { get; } public string Surname { get; } public int Age { get; } public Person(string name, string surname, int age) { Name = name; Surname = surname; Age = age; } }
So adding readonly
removes any possibility of unintended assignment or value change inside or outside an instance of a struct. One thing you need to beware of though, is if you tend to use the parameterless constructor along with property assignment like so
Person s = new Person(); s.Age = 15; s.Name = "asd"; s.Surname = "qwe";
or like the following
Person s = new Person { Age = 15, Name = "asd", Surname = "qwe" };
while the default parameterless constructor for the struct will still be available for you to call, assigning any property will result in a compilation error as the properties are readonly. So any call to the parameterless constructor of the struct, will set the properties to their default values and they will never be changed for the lifetime of the struct instance.
So in conclusion, this will help showing your intent a lot easier as you can define from the start that this struct is immutable and non-editable.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK