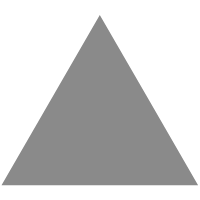
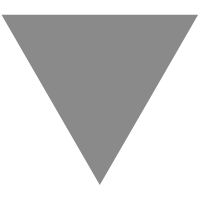
Connecting to MongoDB with NodeJS
source link: https://www.tuicool.com/articles/hit/3IJRF3Z
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
In this article we will learn how we can use and connect to MongoDb with Node.js.
This tutorial is specifically designed for beginners, so if you have a bit of Node.js knowledge and you are just getting started with MongoDB, you are at the right place. This is the MongoDB NodeJS Tutorial for beginners .
MongoDB is a document oriented no sql database. MongoDB stores data in JSON like documents. This gives us the flexibility to have flexible fields in documents and change the data-structure with each document.
We will be using an npm package called as Mongoose which is by far the most popular orm/driver to connect to MongoDB.
In the following sections, I’ll cover the following points.
- Connecting to MongoDB.
- Creating and using a schema in Mongoose.
- Querying MongoDB with Mongoose.
Setting up our environment
Before we start with the actual application, we need to make sure we have a few things installed and running on our machine.
- Install MongoDB . Make sure you follow the installation steps provided on the website clearly, the installation differs depending on your OS.
- Install Node.js . At the time of writing this article 10.15.0 is the latest LTS version.
Once both of these are installed, we need to start our MongoDB. On windows you can run by running mongod. In Linux mongod is added to the services so you can just do sudo service mongod start .
Creating a Node.js Project
We will by creating an empty npm project.
mkdir mongodb-tutorial
Initialize with a package.json.
npm init --yes
Let’s install mongoose.
npm i mongoose --save
Creating db.js. This file will hold the code to connect to mongodb.
touch db.js
db.js
const mongoose = require('mongoose'); const mongoURI = "mongodb://localhost:27017/ciphertrick"; //connecting to ciphertrick const options = { keepAlive: true, keepAliveInitialDelay: 300000, useNewUrlParser: true }; mongoose.connect(mongoURI, options); mongoose.connection.on('connected', ()=>{ console.log('Mongoose default connection open to ' + mongoURI); }); // If the connection throws an error mongoose.connection.on('error', (err)=>{ console.log('handle mongo errored connections: ' + err); }); // When the connection is disconnected mongoose.connection.on('disconnected', ()=>{ console.log('Mongoose default connection disconnected'); }); process.on('SIGINT', ()=>{ mongoose.connection.close(()=>{ console.log('App terminated, closing mongo connections'); process.exit(0); }); });
Mongoose connect function takes the uri and set of options (optional). The URI is comprised of mongodb server and the database name, eg : mongodb://{mongoserver}/{dbname} .
If you are coming from a mysql background, you might be wondering we didn’t even create a database named ciphertrick ? In mongodb you don’t need to create a db before connecting to it, MongoDB does that for us internally, if the database name provided does not exits, MongoDb will create it for us.
In the above file we have added event listeners on various connection events, this is a healthy practice to do, so in case if something goes wrong debugging is easy.
At the end we are closing all db connections when our application terminates.
Now create a file named app.js , this will be the starting point of our app.
app.js
require('./db');
At this point, we are simply requiring our db file.
Let’s run our app and see what we get.
node app.js
If you have followed the tutorial correctly you should get the following output.
Mongoose default connection open to mongodb://localhost:27017/ciphertrick
Creating models and querying MongoDB
A cleaner way is to organize your models into a separate directory.
mkdir models
touch person.js
models/person.js
const mongoose = require("mongoose"); var Schema = new mongoose.Schema({ name: {type:String, required:true}, age: {type: Number, required: true}, gender: {type: String, enum: ['Male', 'Female', 'Other']} }, { timestamps: true}); Schema.statics.addPerson = async function(person){ var Person = new this(person); var result = await Person.save(person); return result; } Schema.statics.listPersons = async function(){ return await this.find(); } module.exports = mongoose.model('person', Schema);
As you can see above, we have defined a model person using mongoose. Defining Mongoose models provides some structure to otherwise unstructured collections. Now one might say MongoDB is made for unstructured data. Well, that is correct and you can also achieve that in here by just passing ‘strict :false ‘ in options. But it is always a plus to have a rough idea how our MongoDB documents look, this makes it easier to interact with them and also helps others understand our code better.
Mongoose also provides inbuilt validations which is a very handy feature.
We have also defined two static methods, one for adding a new person and one for listing persons.
Static functions are directly defined on models and can be invoked by simply requiring the model. It is a good way to organize your functions with the model itself.
If you have read the code above, you will realize how easy it is to interact with MongoDB. It’s just like writing JavaScript. Also if you want to find a specific person by id you can just do…
this.find({_id: "5c4fb96536f7132ad4ccddfe"});
Okay, now that we have understood what we have done and have the code. Let’s just try to call it in our app.js file.
app.js
require('./db'); const Person = require('./models/person'); const test = async function(){ const data = { name: "Jane Doe", age: 24, gender: "Female" } await Person.addPerson(data); const p = await Person.listPersons(); console.log(p); } test();
Run the app..
node app.js
You should have the following output.

In the output you might also, see there are two fields createdAt and updatedAt which we had not defined in the model, that’s because we have timestamps :true set under options. Mongoose adds these fields for us.
Conclusion
So now you know how you can connect to MongoDb using NodeJS. You have also learnt how to do some basic queries.
Hope this MongoDB NodeJS tutorial for beginners will give a good head start and a clear understanding on using Mongoose in your NodeJS projects.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK