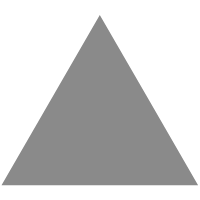
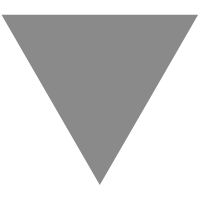
Working With Golang Date and Time
source link: https://www.tuicool.com/articles/hit/fmiye2r
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
This golang tutorial explains how to work with golang time package .The time and date is a very important package for any programming language.
The golang provides time package to measuring and displaying time. You can get current time based on selected timezone, add duration with current timezone etc using go time package.
Table of Contents
- How To Get Current Time in Golang
- How to add Years, Months and Days into Current Time
- How To Add Hours, Minutes, Seconds into Current Time
- How To Get Current UNIX Timestamp in Golang
- How To Parse Date String in Golang
How To Get Current Time in Golang
now := time.Now() fmt.Printf("current time is :%s", now)
The golang time now function outout is:
current time is :2009-11-10 23:00:00 +0000 UTC m=+0.000000001
Date Time in Golang
The date function return the time corresponding yyyy-mm-dd hh:mm:ss + nsec nanoseconds.
date := time.Date(2018, 01, 12, 22, 51, 48, 324359102, time.UTC) fmt.Printf("date is :%s", date)
The golang Date() output is:
date is :2018-01-12 22:51:48.324359102 +0000 UTC
How to add Years, Months and Days into Current Time
The AddDate method help to add Years, Months and Days to corresponding to the date, that could be any date. You can pass integer type value of Years, Months and Days. The return type is time. You can pass any of the three parameters.
date := time.Date(2018, 01, 12, 22, 51, 48, 324359102, time.UTC) next_date := date.AddDate(1, 2, 1) fmt.Printf("date is :%s\n", date) fmt.Printf("next_date is :%s", next_date)
The output is:
date is :2018-01-12 22:51:48.324359102 +0000 UTC next_date is :2019-03-13 22:51:48.324359102 +0000 UTC
How To Add Hours, Minutes, Seconds into Current Time
The golang time package provides add()
method to get future time based on passed value. This method takes integer value of Hours, Minutes, Seconds.
cur_time := time.Now() next_time:= cur_time.Add(time.Hour * 2 + time.Minute * 1+ time.Second * 21) fmt.Printf("current time is :%s\n", cur_time ) fmt.Printf("calculated time is :%s", next_time)
The output is:
current time is :2009-11-10 23:00:00 +0000 UTC m=+0.000000001 calculated time is :2009-11-11 01:01:21 +0000 UTC m=+7281.000000001
How To Get Current UNIX Timestamp in Golang
You can get current time using now()
method, Its have Unix()
method that help to convert time into UNIX timestamp in golang.
cur_time := time.Now().Unix() fmt.Printf("current unix timestamp is :%v\n", cur_time )
The outout is:
current unix timestamp is :1257894000
How To Parse Date String in Golang
You can parse date string in golang using parse method.
date := "2018-10-24T18:50:23.541Z" parse_time, _ := time.Parse(time.RFC3339, date) fmt.Printf("current unix timestamp is :%s\n", date) fmt.Printf("parse_time is :%s", parse_time)
The output is:
current unix timestamp is :2018-10-24T18:50:23.541Z parse_time is :2018-10-24 18:50:23.541 +0000 UTC
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK