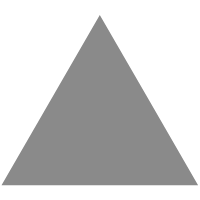
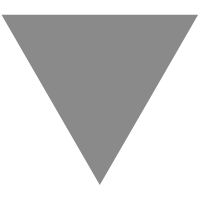
JavaParser: Java Code Generation
source link: https://www.tuicool.com/articles/hit/iuQJFbN
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
In this article, I'm going to show you how to generate Java code using the JavaParser
. I couldn't find much of the documentation available with regards to code generation in javaparser.org or the manual. So, I thought putting this out would help someone who would like to experiment with the Java parser.
In its simplest form, the JavaParser
library allows you to interact with Java source code as a Java object representation in a Java environment. More formally, we refer to this object representation as an Abstract Syntax Tree (AST). Also, it has the ability to manipulate the underlying structure of the source code. This can then be written to a file, providing developers with the facility to build their own code generating software.
First up, you have to instantiate the compilation unit, upon which you will add the remaining pieces of the code.
CompilationUnit compilationUnit = new CompilationUnit();
Then, you can add your import statements to the compilation unit, here:
compilationUnit.addImport("org.springframework.boot.SpringApplication");
You can add your package statement to the compilation unit, as shown below:
compilationUnit.setPackageDeclaration("com.abc.def");
You can add the class declaration to the Java file:
ClassOrInterfaceDeclaration classDeclaration = compilationUnit.addClass("AnyClassName").setPublic(true);
If you want to add annotations at the class level, you can use the following code:
classDeclaration.addAnnotation("AnyAnnotation");
You can add method declarations within your newly declared class, as shown below:
MethodDeclaration methodDeclaration = classDeclaration.addMethod("anyMethodName", PUBLIC); methodDeclaration.setType("AnyReturnType");
You can add arguments to your newly created method declaration:
methodDeclaration.addAndGetParameter(String.class, "args").setVarArgs(true);
Add annotations on top of the newly declared method:
methodDeclaration.addAndGetAnnotation("AnyAnnotation");
To add the method logic/block statement within the newly declared method, use the below code:
BlockStmt blockStmt = new BlockStmt(); methodDeclaration.setBody(blockStmt);
To declare and instantiate a variable within the method/block statement, use the following code:
ExpressionStmt expressionStmt = new ExpressionStmt(); VariableDeclarationExpr variableDeclarationExpr = new VariableDeclarationExpr(); VariableDeclarator variableDeclarator = new VariableDeclarator(); variableDeclarator.setName("anyVariableName"); variableDeclarator.setType(new ClassOrInterfaceType("AnyVariableType")); variableDeclarator.setInitializer("new AnyVariableType()"); NodeList<VariableDeclarator> variableDeclarators = new NodeList<>(); variableDeclarators.add(variableDeclarator); variableDeclarationExpr.setVariables(variableDeclarators); expressionStmt.setExpression(variableDeclarationExpr); blockStmt.addStatement(expressionStmt);
To invoke a method of the new variable created within the method/block statement, use the below code:
NameExpr nameExpr = new NameExpr("anyVariableName"); MethodCallExpr methodCallExpr = new MethodCallExpr(nameExpr, "anyMethodName"); methodCallExpr.addArgument("anyArgument"); blockStmt.addStatement(methodCallExpr);
To return the variable created in the method, use the code below:
ReturnStmt returnStmt = new ReturnStmt(); NameExpr returnNameExpr = new NameExpr(); returnNameExpr.setName("anyVariableName"); returnStmt.setExpression(returnNameExpr); blockStmt.addStatement(returnStmt);
To print the code generated above, just call the toString
method of the compilation unit:
String code = compilationUnit.toString();
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK