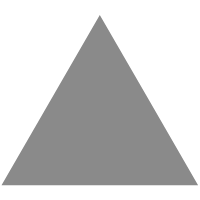
31
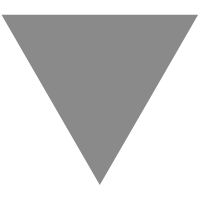
Golang中的一些常用的简单算法
source link: https://studygolang.com/articles/16927?amp%3Butm_medium=referral
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
本文为转载,原文: Golang中的一些常用的简单算法

Golang
介绍
Golang中的一些常用的简单算法, 包括抢红包算法,洗牌算法等
1. 洗牌算法
洗牌算法,即将原来的顺序打乱,组成新的随机排序的顺序。
以下示例中以int切片为例给出一个简单算法:
import ( "fmt" "math/rand" "time" ) func main() { intArr := []int{1,2,3,4,5,6,7,8,9} for i := 0; i < 10; i++{ shuffle(intArr) fmt.Println(intArr) } } // 洗牌算法 func shuffle(arr []int){ rand.Seed(time.Now().UnixNano()) var i, j int var temp int for i = len(arr) - 1; i > 0; i-- { j = rand.Intn(i + 1) temp = arr[i] arr[i] = arr[j] arr[j] = temp } }
测试结果

测试结果
2. 抢红包算法
抢红包算法即类似微信拼手气红包,发一定金额的红包,指定人数抢红包。
以下给出一个简单的算法示例:
import ( "fmt" "math/rand" "time" ) func main() { for i := 0; i < 10; i ++{ redPackage(10, 500) fmt.Println("") } } // 随机红包 // remainCount: 剩余红包数 // remainMoney: 剩余红包金额(单位:分) func randomMoney(remainCount, remainMoney int)int{ if remainCount == 1{ return remainMoney } rand.Seed(time.Now().UnixNano()) var min = 1 max := remainMoney / remainCount * 2 money := rand.Intn(max) + min return money } // 发红包 // count: 红包数量 // money: 红包金额(单位:分) func redPackage(count, money int) { for i := 0; i < count; i++ { m := randomMoney(count - i, money) fmt.Printf("%d ", m) money -= m } }
测试结果:

测试结果
完
本文为原创,转载请注明出处: Golang中的一些常用的简单算法
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK