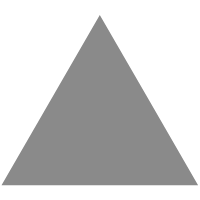
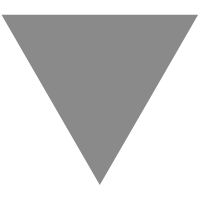
Behavioral Design Patterns: Observer
source link: https://www.tuicool.com/articles/hit/AvmmUza
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
The observer
is one of the most popular design patterns. It has been used on many software use cases and, thus, many languages out there provide it as a part of their standard library.
By using the observer pattern, we can tackle the following challenges.
- Dependency with objects defined in a way that avoids tight coupling
- Changes to an object change its dependent objects
- An object can notify all of its dependent objects
Imagine the scenario of a device with multiple sensors. Some parts of the code will need to get notified when new sensor data arrives and, thus, act accordingly. We will start by looking at a simple class, which represents the JSON data.
package com.gkatzioura.design.behavioural.observer; public class SensorData { private final String sensor; private final Double measure; public SensorData(String sensor, Double measure) { this.sensor = sensor; this.measure = measure; } public String getSensor() { return sensor; } public Double getMeasure() { return measure; } }
Then, we shall create the observer
interface. Every class that implements the observer
interface shall be notified once a new object is created.
package com.gkatzioura.design.behavioural.observer; public interface Observer { void update(SensorData sensorData); }
The next step is to create the observable
interface. The observable
interface will have methods in order to register the observers that need to get notified.
package com.gkatzioura.design.behavioural.observer; public interface Observable { void register(Observer observer); void unregister(Observer observer); void updateObservers(); }
Now, let us add some implementations. The sensor listener will receive data from the sensors and notify the observers about the presence of data.
package com.gkatzioura.design.behavioural.observer; import java.util.ArrayList; import java.util.Iterator; import java.util.List; public class SensorReceiver implements Observable { private List data = new ArrayList(); private List observers = new ArrayList(); @Override public void register(Observer observer) { observers.add(observer); } @Override public void unregister(Observer observer) { observers.remove(observer); } public void addData(SensorData sensorData) { data.add(sensorData); } @Override public void updateObservers() { /** * The sensor receiver has retrieved some sensor data and thus it will notify the observer * on the data it accumulated. */ Iterator iterator = data.iterator(); while (iterator.hasNext()) { SensorData sensorData = iterator.next(); for(Observer observer:observers) { observer.update(sensorData); } iterator.remove(); } } }
Then, we will create an observer
that shall log the sensor data received to the database. It might be an InfluxDB or an elastic search — you name it.
package com.gkatzioura.design.behavioural.observer; public class SensorLogger implements Observer { @Override public void update(SensorData sensorData) { /** * Persist data to the database */ System.out.println(String.format("Received sensor data %s: %f",sensorData.getSensor(),sensorData.getMeasure())); } }
Let’s put it all together.
package com.gkatzioura.design.behavioural.observer; public class SensorMain { public static void main(String[] args) { SensorReceiver sensorReceiver = new SensorReceiver(); SensorLogger sensorLogger = new SensorLogger(); sensorReceiver.register(sensorLogger); sensorReceiver.addData(new SensorData("temperature",1.2d)); sensorReceiver.updateObservers(); } }
You can find the source code on GitHub .
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK