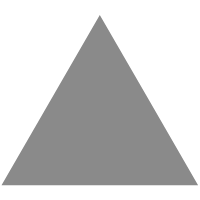
53
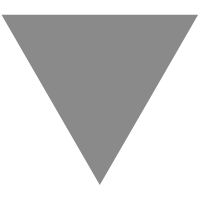
GitHub - kitze/react-hanger: A small collection of useful hooks for React 16.7
source link: https://github.com/kitze/react-hanger
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
README.md
react-hanger
⚠️ Warning: hooks are not part of a stable React release yet, so use this library only for experiments
Install
yarn add react-hanger
Usage
import React, { Component } from "react"; import { useInput, useBoolean, useNumber, useArray, useOnMount, useOnUnmount } from "react-hanger"; const App = () => { const newTodo = useInput(""); const showCounter = useBoolean(true); const limitedNumber = useNumber(3, { lowerLimit: 0, upperLimit: 5 }); const counter = useNumber(0); const todos = useArray(["hi there", "sup", "world"]); const rotatingNumber = useNumber(0, { lowerLimit: 0, upperLimit: 4, rotate: true }); useOnMount(() => console.log("hello world")); useOnUnmount(() => console.log("goodbye world")); return ( <div> <button onClick={showCounter.toggle}> toggle counter </button> <button onClick={counter.increase}> increase </button> {showCounter.value && <span> {counter.value} </span>} <button onClick={counter.decrease}> decrease </button> <button onClick={todos.clear}> clear todos </button> <input type="text" value={newTodo.value} onChange={newTodo.onChangeHandler} /> </div> ); };
Example
useStateful
Just an alternative syntax to useState
, because it doesn't need array destructuring.
It returns an object with value
and a setValue
method.
const username = useStateful("test"); username.setValue("tom"); console.log(username.value);
useOnMount
const App = () => { useOnMount(() => console.log("hello world")); return <div> hello world </div>; };
useOnUnmount
const App = () => { useOnUnmount(() => console.log("goodbye world")); return <div> goodbye world </div>; };
useLifecycleHooks
const App = () => { useLifecycleHooks({ onMount: () => console.log("mounted!"), onUnmount: () => console.log("unmounted!") }); return <div> hello world </div>; };
useBoolean
const showCounter = useBoolean(true);
Methods:
toggle
setTrue
setFalse
useNumber
const counter = useNumber(0); const limitedNumber = useNumber(3, { upperLimit: 5, lowerLimit: 3 }); const rotatingNumber = useNumber(0, { upperLimit: 5, lowerLimit: 0, rotate: true });
Methods:
increase
decrease
Options:
lowerLimit
upperLimit
rotate
useInput
const newTodo = useInput("");
<input value={newTodo.value} onChange={newTodo.onChangeHandler} />
<input {...newTodo.bindToInput} />
Methods:
clear
onChangeHandler
bindToInput
Properties:
hasValue
useArray
const todos = useArray([]);
Methods:
add
clear
removeIndex
removeById
useSetState
const { state, setState } = useSetState({ loading: false }); setState({ loading: true, data: [1, 2, 3] });
Methods:
setState(value)
- will merge thevalue
with the currentstate
(like this.setState works in React)
Properties:
state
- the current state
usePrevious
Use it to get the previous value of a prop or a state value.
It's from the official React Docs.
It might come out of the box in the future.
const Counter = () => { const [count, setCount] = useState(0); const prevCount = usePrevious(count); return ( <h1> Now: {count}, before: {prevCount} </h1> ); };
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK