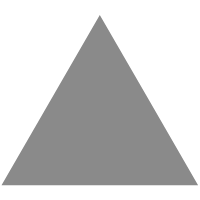
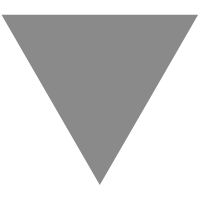
GitHub - palmerhq/the-platform: Web. Components.
source link: https://github.com/palmerhq/the-platform
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
README.md
The Platform
Browser API's turned into React Hooks and Suspense-friendly React elements for common situations.
Install
npm i the-platform
API
Hooks
useDeviceMotion()
Detect and retrieve current device Motion.
Returns
Example
import { useDeviceMotion } from 'the-platform'; const Example = () => { const { acceleration, rotationRate, interval } = useDeviceMotion(); // ... };
useDeviceOrientation()
Detect and retrieve current device orientation.
Returns
Example
import { useDeviceOrientation } from 'the-platform'; const Example = () => { const { alpha, beta, gamma, absolute } = useDeviceOrientation(); // ... };
useGeoPosition()
Retrieve Geo position from the browser. This will throw a promise (must use with Suspense).
Arguments
Returns
Example
import { useGeoPosition } from 'the-platform'; const Example = () => { const { coords: { latitude, longitude }, } = useGeoPosition(); // ... };
useNetworkStatus()
Retrieve network status from the browser.
Returns
Object containing:
-
online: boolean
:true
if the browser has network access.false
otherwise. -
offlineAt?: Date
: Date when network connection was lost.
Example
import { useNetworkStatus } from 'the-platform'; const Example = () => { const { online, offlineAt } = useNetworkStatus(); // ... };
useMedia()
Arguments
query: string | object
: media query string or object (parsed by json2mq).
Returns
match: boolean
: true
if the media query matches, false
otherwise.
Example
import { useMedia } from 'the-platform'; const Example = () => { const small = useMedia('(min-width: 400px)'); const medium = useMedia({ minWidth: 800 }); // ... };
useScript()
This will throw a promise (must use with Suspense).
Arguments
Object containing:
src: string
: The script's URI.
import { useScript } from 'the-platform'; const Example = () => { const _unused = useScript({ src: 'bundle.js' }); // ... };
useStylesheet()
This will throw a promise (must use with Suspense).
Arguments
Object containing:
href: string
: The stylesheet's URI.media?: string
: Intended destination media for style information.
import { useStylesheet } from 'the-platform'; const Example = () => { const _unused = useStylesheet({ href: 'normalize.css' }); // ... };
useWindowScrollPosition()
Returns
Object containing:
x: number
: Horizontal scroll in pixels (window.pageXOffset
).y: number
: Vertical scroll in pixels (window.pageYOffset
).
Example
import { useWindowScrollPosition } from 'the-platform'; const Example = () => { const { x, y } = useWindowScrollPosition(); // ... };
useWindowSize()
Returns
Object containing:
width
: Width of browser viewport (window.innerWidth
)height
: Height of browser viewport (window.innerHeight
)
Example
import { useWindowSize } from 'the-platform'; const Example = () => { const { width, height } = useWindowSize(); // ... };
Components
<Img>
Props
src: string
- anything else you can pass to an
<img>
tag
import React from 'react'; import { Img } from 'the-platform'; function App() { return ( <div> <h1>Hello</h1> <React.Suspense maxDuration={300} fallback={'loading...'}> <Img src="https://source.unsplash.com/random/4000x2000" /> </React.Suspense> </div> ); } export default App;
<Script>
Props
src: string
children?: () => React.ReactNode
- This render prop will only execute after the script has loaded.- anything else you can pass to a
<script>
tag
import React from 'react'; import { Script } from 'the-platform'; function App() { return ( <div> <h1>Load Stripe.js Async</h1> <React.Suspense maxDuration={300} fallback={'loading...'}> <Script src="https://js.stripe.com/v3/" async> {() => console.log(window.Stripe) || null} </Script> </React.Suspense> </div> ); } export default App;
<Video>
Props
src: string
- anything else you can pass to a
<video>
tag
import React from 'react'; import { Video } from 'the-platform'; function App() { return ( <div> <h1>Ken Wheeler on a Scooter</h1> <React.Suspense maxDuration={300} fallback={'loading...'}> <Video src="https://video.twimg.com/ext_tw_video/1029780437437014016/pu/vid/360x640/QLNTqYaYtkx9AbeH.mp4?tag=5" preload="auto" autoPlay /> </React.Suspense> </div> ); } export default App;
<Audio>
Props
src: string
- anything else you can pass to a
<audio>
tag
import React from 'react'; import { Audio } from 'the-platform'; function App() { return ( <div> <h1>Meavy Boy - Compassion</h1> {/* source: http://freemusicarchive.org/music/Meavy_Boy/EP_71_to_20/Compassion */} <React.Suspense maxDuration={300} fallback={'loading...'}> <Audio src="https://file-dnzavydoqu.now.sh/" preload="auto" autoPlay /> </React.Suspense> </div> ); } export default App;
<Preload>
Preload a resource with <link rel="preload">
. For more information check out MDN or the Google Developer Blog.
Props
href: string
as: string
- resource type
import React from 'react'; import { Preload, Script } from 'the-platform'; function App() { return ( <div> <h1>Preload</h1> <React.Suspense maxDuration={300} fallback={'loading...'}> <Preload href="https://js.stripe.com/v3/" rel="preload" as="script" /> <Script src="https://js.stripe.com/v3/" async /> </React.Suspense> </div> ); } export default App;
<Stylesheet>
Lazy load a stylesheet.
Props
href: string
import React from 'react'; import { Stylesheet } from 'the-platform'; function App() { return ( <div> <h1>Styles</h1> <React.Suspense maxDuration={300} fallback={'loading...'}> <Stylesheet href="style.css" /> </React.Suspense> </div> ); } export default App;
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK