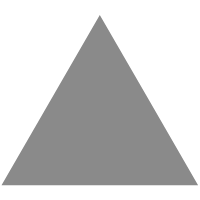
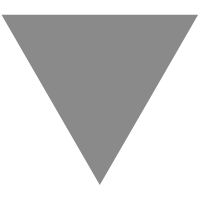
Headless CMS and Decoupled CMS in .NET Core
source link: https://www.tuicool.com/articles/hit/riUBZne
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
I'm sure I'll miss some, so if I do, please sound off in the comments and I'll update this post over the next week or so!
Lately I've been noticing a lot of "Headless" CMSs (Content Management System). A ton, in fact. I wanted to explore this concept and see if it's a fad or if it's really something useful.
Given the rise of clean RESTful APIs has come the rise of Headless CMS systems. We've all evaluated CMS systems (ones that included both front- and back-ends) and found the front-end wanting. Perhaps it lacks flexibility OR it's way too flexible and overwhelming. In fact, when I wrote my podcast website I considered a CMS but decided it felt too heavy for just a small site.
A Headless CMS is a back-end only content management system (CMS) built from the ground up as a content repository that makes content accessible via a RESTful API for display on any device.
I could start with a database but what if I started with a CMS that was just a backend - a headless CMS. I'll handle the front end, and it'll handle the persistence.
Here's what I found when exploring .NET Core-based Headless CMSs. One thing worth noting, is that given Docker containers and the ease with which we can deploy hybrid systems, some of these solutions have .NET Core front-ends and "who cares, it returns JSON" for the back-end!
Lynicon
Lyncicon is literally implemented as a NuGet Library ! It stores its data as structured JSON. It's built on top of ASP.NET Core and uses MVC concepts and architecture.
It does include a front-end for administration but it's not required. It will return HTML or JSON depending on what HTTP headers are sent in. This means you can easily use it as the back-end for your Angular or existing SPA apps.
Lyncion is largely open source at https://github.com/jamesej/lyniconanc . If you want to take it to the next level there's a small fee that gives you updated searching, publishing, and caching modules.
ButterCMS
ButterCMS is an API-based CMS that seamlessly integrates with ASP.NET applications. It has an SDK that drops into ASP.NET Core and also returns data as JSON. Pulling the data out and showing it in a few is easy.
public class CaseStudyController : Controller { private ButterCMSClient Client; private static string _apiToken = ""; public CaseStudyController() { Client = new ButterCMSClient(_apiToken); } [Route("customers/{slug}")] public async Task<ActionResult> ShowCaseStudy(string slug) { butterClient.ListPageAsync() var json = await Client.ListPageAsync("customer_case_study", slug) dynamic page = ((dynamic)JsonConvert.DeserializeObject(json)).data.fields; ViewBag.SeoTitle = page.seo_title; ViewBag.FacebookTitle = page.facebook_open_graph_title; ViewBag.Headline = page.headline; ViewBag.CustomerLogo = page.customer_logo; ViewBag.Testimonial = page.testimonial; return View("Location"); } }
Then of course output into Razor (or putting all of this into a RazorPage) is simple:
<html> <head> <title>@ViewBag.SeoTitle</title> <meta property="og:title" content="@ViewBag.FacebookTitle" /> </head> <body> <h1>@ViewBag.Headline</h1> <img width="100%" src="@ViewBag.CustomerLogo"> <p>@ViewBag.Testimonial</p> </body> </html>
Butter is a little different (and somewhat unusual) in that their backend API is a SaaS (Software as a Service) and they host it. They then have SDKs for lots of platforms including .NET Core. The backend is not open source while the front-end is https://github.com/ButterCMS/buttercms-csharp .
Piranha CMS
Piranha CMS is built on ASP.NET Core and is open source on GitHub . It's also totally package-based using NuGet and can be easily started up with a dotnet new template like this:
dotnet new -i Piranha.BasicWeb.CSharp dotnet new piranha dotnet restore dotnet run
It even includes a new Blog template that includes Bootstrap 4.0 and is all set for customization. It does include optional lightweight front-end but you can use those as guidelines to create your own client code. One nice touch is that Piranha also images image resizing and cropping.
Umbraco Headless
The main ASP.NET website currently uses Umbraco as its CMS. Umbraco is a well-known open source CMS that will soon include a Headless option for more flexibility. The open source code for Umbraco is up here https://github.com/umbraco .
Orchard Core
Orchard is a CMS with a very strong community and fantastic documentation . Orchard Core is a redevelopment of Orchard using open source ASP.NET Core. While it's not "headless" it is using a Decoupled Architecture . Nothing would prevent you from removing the UI and presenting the content with your own front-end. It's also cross-platform and container friendly.
Squidex
"Squidex is an open source headless CMS and content management hub. In contrast to a traditional CMS Squidex provides a rich API with OData filter and Swagger definitions." Squidex is build with ASP.NET Core and the CQRS pattern and works with both Windows and Linux on today's browsers.
Squidex is open source with excellent docs at https://docs.squidex.io . Docs are at https://docs.squidex.io . They are also working on a hosted version you can play with here https://cloud.squidex.io . Samples on how to consume it are here https://github.com/Squidex/squidex-samples .
The consumption is super clean:
[Route("/{slug},{id}/")] public async Task<IActionResult> Post(string slug, string id) { var post = await apiClient.GetBlogPostAsync(id); var vm = new PostVM { Post = post }; return View(vm); }
And then the View:
@model PostVM @{ ViewData["Title"] = Model.Post.Data.Title; } <div> <h2>@Model.Post.Data.Title</h2> @Html.Raw(Model.Post.Data.Text) </div>
What .NET Core Headless CMSs did I miss? Let me know.
*Photo "headless" by Wendy used under CC https://flic.kr/p/HkESxW
Sponsor: Telerik DevCraft Telerik DevCraft is the comprehensive suite of .NET and JavaScript components and productivity tools developers use to build high-performant, modern web, mobile, desktop apps and chatbots. Try it!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK