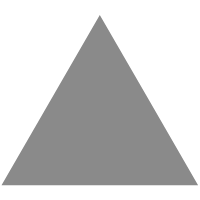
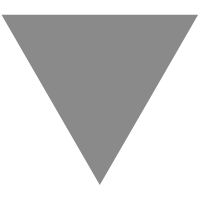
Using Airtable as a Handy Cloud Storage for your Apps
source link: https://www.tuicool.com/articles/hit/JramQ3N
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Using Airtable as a Handy Cloud Storage for your Apps

What is Airtable
Airtable is an online service that you can use as a very convenient backend storage option.
Why is it convenient? Because:
- you don’t have to manage a server, set up authentication, and keep it secure.
- you can access the data at any time and edit it through a nice interface.
The Airtable company provides the basic service for free, with some limitations you need to be aware of.
Shared in Bit’s blog :star:️
Bit turns components into building blocks which your team can share, discover and use anywhere to build amazing apps faster. Give it a try.
What are the limits?
The API has a limit of 5 requests per second, which is not high, but still reasonable to work with for most scenarios.
Using the Airtable Node.js library which I’ll introduce later on, you don’t have to worry about handling those rate limits yourself, since it comes with built-in logic to handle them, plus an automatic repeat of any rejected request.
The free plan has a limit of 1200 records per each base, which means across all the base tables. Paid plans allow more entries.
The nice API documentation
One of the things you’ll likely appreciate the most as a developer is a good API documentation.
AirTable has a very useful documentation and one of the those I like to point out when it comes to having a great example of docs for developers.
The examples are many and the parameters you can pass are all well documented.
They provide a set of URL and Node.js examples, and all of those are based on the actual data you have in your tables.
Also, any API call parameter set already comes with your API keys and tables and view names pre-filled. It’s great because you don’t have to look at a random example and switch context, and you can work with your own data.
The Airtable terminology
When working with Airtable you’ll find some terms familiar if you used databases before, and some unique to Airtable.
A base is a database, a set of tables related to your application.
A table is one set of data, organized in columns
A view is a particular representation of a table. You can have the same data presented in different views.
You always reference one view, one table, and one base to fetch your data.
Tip for SQL users: a view is not what you are used to; every table has at least one view.
Create a base on Airtable
Once you create an Airtable account you can see the list of sample bases. You can create a new base by selecting a workspace, and clicking “Add a base”:

You’ll be presented with a list of choices.

You can start with a template, and Airtable provides a lot of different templates to choose from:

You can also import an existing spreadsheet, or start from scratch, which is what we’ll do now.

Choose a color and an icon:

And clicking the base icon will open the table view. Here you have the visualization of your “My base” base , showing the “Table 1” table , with the “Grid view” view :

Edit the table fields
From here, clicking the down arrow at the right of each column gives you many choices. Use “Rename field” and “Customize field type” to create the table structure you want.

Every field has a type. You can choose from many predefined fields, which should satisfy most of your needs:

Enough with the description of Airtable as a product. Let’s see how to work with its API.
For the sake of following the examples, let’s keep the default column names and types.
Working with the API using the Node.js official client library
Airtable provides an official Node.js library, available on npm
as airtable
. Install it in your app using
npm install airtable
Once the library is installed, you can use it in a Node.js app
const Airtable = require('airtable');
Airtable.configure({ apiKey: process.env.API_KEY });
where API_KEY is an environment variable you configure before running the program.
As you work with your API, you are likely to use other variables that set the name of a base, the name of a table, and the name of a view:
const BASE_NAME = ''; const TABLE_NAME = ''; const VIEW_NAME = '';
Use those to initialize a base, and to fetch a table reference:
const base = Airtble.base(BASE_NAME); const table = base(TABLE_NAME);
The VIEW_NAME variable will be used when selecting elements from a table, using the table.select()
method.
CRUD (Create Read Update Delete)
Let’s now see how to perform some very common data operations.
Create a record
You can add a new record by calling the create()
method on the table object. You pass an object with the field names (you don’t need to pass all the fields, and the ones missing will be kept empty).
In this case, if there is an error we just log it, and then we output the id
of the row just entered.
table.create({ "Name": "My pet", "Notes": "My pet is a dog" }, (err, record) => { if (err) { console.error(err); return; }
console.log(record.getId()); });
Read a record
You can get a specific record by using the find()
method on the table object, passing a record id:
const id = '/* the record id */';
table.find(id, (err, record) => { if (err) { console.error(err); return; }
/* here we have the record object we can inspect */ console.log(record); });
From the record object returned by find()
we can get the record content:
record.get('Name'); record.get('Notes');
You can get the record id using record.getId()
and you can get the time the record was created using record.createdTime
.
Update a record
Update one or more fields of a record, using the update
method of the table object:
const id = '/* the record id */';
table.update(id, { "Name": "My car" }, (err, record) => { if (err) { console.error(err); return; }
console.log(record.get('Name')); //"My car" });
In this case, fields not mentioned are not touched: they are left with whatever value they were before.
To clean the unlisted fields, use the replace()
method:
const id = '/* the record id */';
table.replace(id, { "Name": "My car", }, (err, record) => { if (err) { console.error(err); return; }
//... })
Delete a record
To delete a record from the table, call the destroy()
method of the table object:
const id = '/* the record id */';
table.destroy(id, (err, record) => { if (err) { console.error(err); return; }
console.log('Deleted record'); });
Get the list of records
So far we’ve seen how to operate with a single record object. How can you iterate upon all the records in a table? Using the select
method of the table object:
table.select({ view: VIEW_NAME }).firstPage((err, records) => { if (err) { console.error(err); return; }
//you now have access to the records array });
notice we used the firstPage()
method. This gives us access to the first page of records, which by default shows the first 100 items.
Pagination
If you do have more than 100 items in your table, to get access to the other records you have to use the pagination functionality. Instead of calling firstPage()
you call eachPage()
, which accepts 2 functions as arguments.
The first, processPage()
, is a function that is called upon every page of records. In there, we simply add the page records (available through the first parameter, filled automatically by the Airtable API).
We add those partial set to the allRecords
array, and we call the fetchNextPage()
function to continue fetching the other records.
The processAllRecords()
function is called when all the records have been successfully fetched. There is one single parameter here, which contains an error object if there is an error, otherwise, we are safe to use the allRecords
array values.
let allRecords = [];
const processPage = (pageRecords, fetchNextPage) => { records = [...allRecords, ...pageRecords]; fetchNextPage(); };
const processAllRecords = err => { if (err) { console.error(err); return; }
//we now have all the records in allRecords console.log(allRecords) };
table.select({ view: VIEW_NAME }).eachPage(processPage, processAllRecords);
Closing words
Airtable is a useful service to use for building prototypes and to build small applications that need a fair amount of data.
It’s great to be able to see the data and edit it through a well-thought user interface. It’s especially nice if you have non-technical people that need to interact with that data.
The free service allows up to 1200 records per base, with paid plans you can get up to 5000 if you have the Plus plan, and 50.000 records with the Pro plan, and more using the enterprise plan.
Feel free to comment below, suggest idea or ask anything! thanks :smiley:
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK