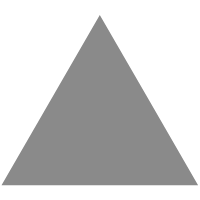
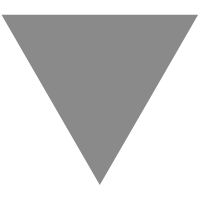
ReactNative学习笔记(四)之自定义文本组件与Image组件
source link: https://mundane799699.github.io/2018/09/08/react-native-note4/?amp%3Butm_medium=referral
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
接上一篇 ReactNative学习笔记(三)之flexbox 继续学习ReactNative
自定义文本组件
类似于Android中的自定义View, 其实就是自定义一个React组件, 用一个class, 后面是组件名字, 继承自React.Component, 里面是一个render方法, 具体代码如下:
class HeaderText extends Component { render() { return ( <Text style={styles.itemText}> {this.props.children} </Text> ); } }
其实this.props.children指的是标签中间的文字.
然后我们应用这个HeaderText
export default class App extends Component<Props> { render() { return ( <View style={styles.container}> <HeaderText> ninghao.net </HeaderText> </View> ); } }
运行看一下效果

可以看到这里面的文字样式就是在HeaderText中定义的styles.itemText样式
Image
Image组件是用来显示图片的, 可以显示本地图像也可以显示网络图像.
首先我们需要引入Image这个组件
import {Platform, StyleSheet, Text, View, Image} from 'react-native';
然后使用Image这个组件并且指定图像的地址
export default class App extends Component<Props> { render() { return ( <View style={styles.container}> <Image style={styles.image} source={{uri: 'https://img3.doubanio.com/view/photo/l/public/p2191398861.jpg'}}> </Image> </View> ); } }
需要注意的是只有我们设置了图像的宽高, 图片才能显示出来
let styles = StyleSheet.create({ image: { width: 99, height: 138, margin: 6, }, ... });
运行效果

ImageBackground
最新版的ReactNative出了ImageBackground这样一个组件, 并且 不允许在Image组件下放置children了 .我们可以去使用这个ImageBackground组件去显示背景.
首先还是需要先导入
import {Platform, StyleSheet, Text, View, Image, ImageBackground} from 'react-native';
然后在代码中使用
export default class App extends Component<Props> { render() { return ( <View style={styles.container}> <ImageBackground style={styles.backgroundImage} source={{uri: 'https://img3.doubanio.com/view/photo/l/public/p2191398861.jpg'}}> <View style={styles.overlay}> <Text style={styles.overlayHeader}> 机器人总动员 </Text> <Text style={styles.overlaySubHeader}> Wall . E ( 2008 ) </Text> </View> </ImageBackground> </View> ); } }
下面是具体的属性值
let styles = StyleSheet.create({ overlay: { backgroundColor: 'rgba(0, 0, 0, 0.1)', alignItems: 'center', }, overlayHeader: { fontSize: 33, fontFamily: 'Helvetica Neue', fontWeight: '200', color: '#eae7ff', padding: 10, }, overlaySubHeader: { fontSize: 16, fontFamily: 'Helvetica Neue', fontWeight: '200', color: '#eae7ff', padding: 10, paddingTop: 0, }, backgroundImage: { flex: 1, resizeMode: 'cover', // contain, stretch(拉伸) }, ... });
rgba表示带透明通道使用rgb表示的颜色.
运行效果:

很漂亮, 不是吗?
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK