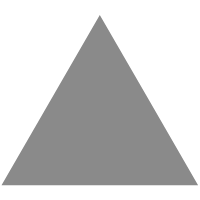
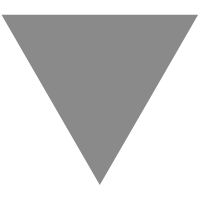
Turning Express and Koa into Function Oriented Servers
source link: https://www.tuicool.com/articles/hit/Fv6BZvY
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Sometimes you may want to expose an existing JavaScript API to clients. You may or may not have architectural reasons and this article will not go into those by debating the pros and cons on REST based vs RPC based approaches. There are plenty or articles on the web about this, just Google.
First, I provide a few details about why not JSON-RPC, then the two lines of code required to enable Express or Koa are shown. Other than that, you can expose your APIs with just one line of code per function. If you have an object that already has the functions on it, you can just pass it in!
Why Not JSON-RPC
JSON-RPC is a fine, simple, industry standard protocol. I didn’t want to go down the JSON-RPC route for the same reason I did not want to go down the REST route. I simply wanted to be able to expose an already existing, well-defined, and well-understood API without having to create a whole new set of documentation, build volumes of unit tests, or run the risk of changing the API semantics during the mapping/wrapping process. In short, I wanted to be efficient for myself and others. FOS ( introduced in an earlier article ) does this with the exception of two things,:
- Functions exposed to clients are somewhat unavoidably asynchronous.
- Errors are somewhat buried from clients. FOS is currently in an ALPHA state and this will be addressed in a subsequent release were the errors will optionally be returned to the client and thrown just like they were on the server (with or without a call stack).
FOS even handles passing those pesky values unsupported by JSON: Infinity
, NaN
and undefined
. It will event allow server functions to return other functions to clients.
Enabling Express and KOA
Examples seem best here. The FOS below simply echos a value after upper casing it.
const express = require('express'), fosify = require("../index.js").fosify, app = express();
fosify(app, {echo:arg => arg,upper:arg => arg.toUpperCase()}, // permit running on any client // mount the functions on the variable F {allow:"*",name:"F"}); app.use(express.static(__dirname + "/")); app.listen(3000, () => console.log("Express FOS listening on 3000"))
or
const Koa = require('koa'), fosify = require("../index.js").fosify, app = new Koa();
app.use(require('koa-static')(__dirname + "/")); fosify(app, {echo:arg => arg,upper:arg => arg.toUpperCase()}, {allow:"*",name:"F"}); app.listen(3000,() => console.log("Koa FOS listening on 3000"));
The HTML file below will download the FOS client and call the server function:
<html> <head> <script src="http://localhost:3000/fos"></script> </head> <body> <script> F.echo("a").then(result => alert(result)); </script> </body> </html>
So, the two lines of code are the import
statement and the fosify
call around an Express or Koa app. Under the covers, this simply adds a special route to the app. The rest of the code is up to you. You can add as many functions as you wish to the object that is the second argument to fosify
. The full signature is:
fosify(app,functions,{allow,name,before,after,done}={})
app — The Express or Koa app
functions — An object, the key names of which are function names and the values of which are the functions. Nested objects are fine and dot notation can be used on the client. If you have an object that already has the functions on it, you can just pass it in!
allow — Provides the same functionality as the allow header in HTTP requests.
name — The name of the client side variable to use. It is also possible to serve up the functions in an anonymous manner as documented in the GitHub README.md .
before, after, and done — Also see the README.md.
Wrap Up
I hope you see the value of FOS. Is has dramatically sped up some of my coding efforts. Give this article a clap if you think it might do the same for you.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK