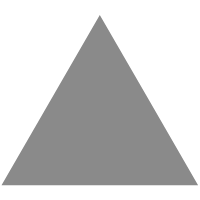
46
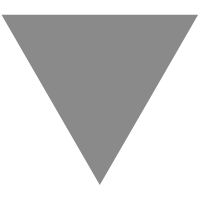
GitHub - fast-average-color/fast-average-color: Fast Average Color...
source link: https://github.com/fast-average-color/fast-average-color
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
README.md
Fast Average Color
A simple library that calculates the average color of any images or videos in browser environment.
Examples
- Background
- Box shadow
- Box shadow, 4 sides
- Border
- Gallery
- Gradient
- Gradient as stripes
- Canvas
- Text photo
- Ambilight
- Define the average color for your images
Using
npm i -D fast-average-color
Simple
<html> <body> ... <div class="image-container"> <img src="image.png" /> <div> Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. </div> </div> <script src="https://unpkg.com/fast-average-color/dist/index.min.js"></script> <script> window.addEventListener('load', function() { var fac = new FastAverageColor(), container = document.querySelector('.image-container'), color = fac.getColor(container.querySelector('img')); container.style.backgroundColor = color.rgba; container.style.color = color.isDark ? '#fff' : '#000'; console.log(color); // { // error: null, // rgb: 'rgb(255, 0, 0)', // rgba: 'rgba(255, 0, 0, 1)', // hex: '#ff0000', // hexa: '#ff0000ff', // value: [255, 0, 0, 255], // isDark: true, // isLight: false // } }, false); </script> </body> </html>
or
<html> <body> ... <div class="image-container"> <img src="image.png" /> <div> Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. </div> </div> <script src="https://unpkg.com/fast-average-color/dist/index.min.js"></script> <script> var fac = new FastAverageColor(), container = document.querySelector('.image-container'); fac.getColorAsync(container.querySelector('img'), function(color) { container.style.backgroundColor = color.rgba; container.style.color = color.isDark ? '#fff' : '#000'; }); </script> </body> </html>
CommonJS
'use strict'; const FastAverageColor = require('fast-average-color'); const fac = new FastAverageColor(); const color = fac.getColor(document.querySelector('img')); console.log(color);
ES6
import FastAverageColor from 'fast-average-color/dist/index.es6'; const fac = new FastAverageColor(); const color = fac.getColor(document.querySelector('img')); console.log(color);
API
.getColor(resource, [options])
/** * Get synchronously the average color from images, videos and canvas. * * @param {HTMLImageElement|HTMLVideoElement|HTMLCanvasElement} resource * @param {Object|null} [options] * @param {Array} [options.defaultColor=[255, 255, 255, 255]] * @param {*} [options.data] * @param {string} [options.mode="speed"] "precision" or "speed" * @param {string} [options.algorithm="sqrt"] "simple" or "sqrt" * @param {number} [options.step=1] * @param {number} [options.left=0] * @param {number} [options.top=0] * @param {number} [options.width=width of resource] * @param {number} [options.height=height of resource] * * @returns {Object} */
Get the average color from a resource (loaded images, videos or canvas).
const fac = new FastAverageColor(); let color; // From loaded image (HTMLImageElement) color = fac.getColor(image); // From loaded image with default color color = fac.getColor({ // Set default color - red. defaultColor: [255, 0, 0, 255] }); // From loaded image with precision color = fac.getColor({ // Modes: 'speed' (by default) or 'precision'. // Current mode is precision. mode: 'precision' }); // From canvas (HTMLCanvasElement) color = fac.getColor(canvas); // From video (HTMLVideoElement) color = fac.getColor(video);
.getColorAsync(resource, callback, [options])
/** * Get asynchronously the average color from not loaded image. * * @param {HTMLImageElement} resource * @param {Function} callback * @param {Object|null} [options] * @param {Array} [options.defaultColor=[255, 255, 255, 255]] * @param {*} [options.data] * @param {string} [options.mode="speed"] "precision" or "speed" * @param {string} [options.algorithm="sqrt"] "simple" or "sqrt" * @param {number} [options.step=1] * @param {number} [options.left=0] * @param {number} [options.top=0] * @param {number} [options.width=width of resource] * @param {number} [options.height=height of resource] */
Get asynchronously the average color from a resource (not loaded images, videos or canvas).
const fac = new FastAverageColor(); // From not loaded image (HTMLImageElement) fac.getColorAsync(image1, function(color) { console.log(color); // { // error: null, // rgb: 'rgb(255, 0, 0)', // rgba: 'rgba(255, 0, 0, 1)', // hex: '#ff0000', // hexa: '#ff0000ff', // value: [255, 0, 0, 255], // isDark: true, // isLight: false // } }); // Advanced example fac.getColorAsync(image2, function(color, data) { console.log(this); // this = image2 console.log(color); // { // error: null, // rgb: 'rgb(255, 0, 0)', // rgba: 'rgba(255, 0, 0, 1)', // hex: '#ff0000', // hexa: '#ff0000ff', // value: [255, 0, 0, 255], // isDark: true, // isLight: false // } console.log(data); // { // myProp: 1 // } }, { // red 0-255, green 0-255, blue 0-255, alpha 0-255 defaultColor: [255, 100, 100, 200], data: { myProp: 1 } });
.getColorFromArray4(array, options)
/** * Get the average color from a array when 1 pixel is 4 bytes. * * @param {Array|Uint8Array} arr * @param {Object} [options] * @param {string} [options.algorithm="sqrt"] "simple" or "sqrt" * @param {Array} [options.defaultColor=[255, 255, 255, 255]] * @param {number} [options.step=1] * * @returns {Array} [red (0-255), green (0-255), blue (0-255), alpha (0-255)] */
Get the average color from a array when 1 pixel is 4 bytes.
const fac = new FastAverageColor(); const buffer = [ // red, green, blue, alpha 200, 200, 200, 255, 100, 100, 100, 255 ]; const color = fac.getColorFromArray4(buffer); console.log(color); // [150, 150, 150, 255]
.destroy()
const fac = new FastAverageColor(); const color = fac.getColor(document.querySelector('img')); //... // The instance is no longer needed. fac.destroy();
Different Builds
In the dist/
directory of the NPM package you will find many different builds.
index.js
Production
index.min.js
ES6
index.es6.js
Development
git clone [email protected]:fast-average-color/fast-average-color.git ./fast-average-color
cd ./fast-average-color
npm i
npm test
open ./examples/
License
MIT License
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK