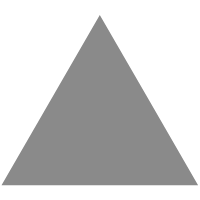
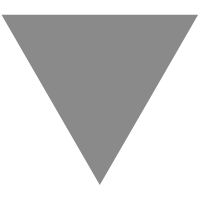
D3.js Tutorial: Building Interactive Bar Charts
source link: https://www.tuicool.com/articles/hit/7Fn67fI
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Recently, we had the pleasure to participate in a machine learning project that involves libraries like React and D3.js. Among many tasks, I developed few charts that help to process the result of ML models like Naive Bayes in form of a line chart or grouped bar chart.
In this article, I would like to present my progress with D3.js so far and show the basic usage of the library through the simple example of a bar chart.
After reading this article, you’ll learn how to create D3.js charts like this easily:
The full source code is available here .
We at RisingStack are fond of the JavaScript ecosystem, backend, and front-end development as well. Personally, I am interested in both of them. On the backend, I can see through the underlying business logic of an application while I also have the opportunity to create awesome looking stuff on the front-end. That’s where D3.js comes into the picture!
What is D3.js?
D3.js is a data-driven JavaScript library for manipulating DOM elements.
“D3 helps you bring data to life using HTML, SVG, and CSS. D3’s emphasis on web standards gives you the full capabilities of modern browsers without tying yourself to a proprietary framework, combining powerful visualization components and a data-driven approach to DOM manipulation.” - d3js.org
Why would You create charts with D3.js in the first place? Why not just display an image?
Well, charts are based on information coming from third-party resources which requires dynamic visualization during render time. Also, SVG is a very powerful tool which fits well to this application case.
Let’s take a detour to see what benefits we can get from using SVG.
The benefits of SVG
SVG stands for Scalable Vector Graphics which is technically an XML based markup language.
It is commonly used to draw vector graphics, specify lines and shapes or modify existing images. You can find the list of available elements here .
Pros:
- Supported in all major browsers;
- It has DOM interface, requires no third-party lib;
- Scalable, it can maintain high resolution;
- Reduced size compared to other image formats.
Cons:
- It can only display two-dimensional images;
- Long learning curve;
- Render may take long with compute-intensive operations.
Despite its downsides, SVG is a great tool to display icons, logos, illustrations or in this case, charts.
Getting started with D3.js
I picked bar chart to get started because it represents a low complexity visual element while it teaches the basic application of D3.js itself. This should not deceive You, D3 provides a great set of tools to visualize data. Check out its github page for some really nice use cases!
A bar chart can be horizontal or vertical based on its orientation. I will go with the vertical one aka Column chart.
On this diagram, I am going to display the top 10 most loved programming languages based on Stack Overflow’s 2018 Developer Survey result .
Time to draw!
SVG has a coordinate system that starts from the top left corner (0;0). Positive x-axis goes to the right, while the positive y-axis heads to the bottom. Thus, the height of the SVG has to be taken into consideration when it comes to calculating the y coordinate of an element.
That’s enough background check, let’s write some code!
I want to create a chart with 1000 pixels width and 600 pixels height.
<body> <svg /> </body> <script> const margin = 60; const width = 1000 - 2 * margin; const height = 600 - 2 * margin; const svg = d3.select('svg'); </script>
In the code snippet above, I select the created <svg>
element in the HTML file with d3 select
. This selection method accepts all kind of selector strings and returns the first matching element. Use selectAll
if You would like to get all of them.
I also define a margin value which gives a little extra padding to the chart. Padding can be applied with a <g>
element translated by the desired value. From now on, I draw on this group to keep a healthy distance from any other contents of the page.
const chart = svg.append('g') .attr('transform', `translate(${margin}, ${margin})`);
Adding attributes to an element is as easy as calling the attr
method. The method’s first parameter takes an attribute I want to apply to the selected DOM element. The second parameter is the value or a callback function that returns the value of it. The code above simply moves the start of the chart to the (60;60) position of the SVG.
Supported D3.js input formats
To start drawing, I need to define the data source I’m working from. For this tutorial, I use a plain JavaScript array which holds objects with the name of the languages and their percentage rates but it’s important to mention that D3.js supports multiple data formats.
The library has built-in functionality to load from XMLHttpRequest, .csv files, text files etc. Each of these sources may contain data that D3.js can use, the only important thing is to construct an array out of them. Note that, from version 5.0 the library uses promises instead of callbacks for loading data which is a non-backward compatible change.
Scaling, Axes
Let’s go on with the axes of the chart. In order to draw the y-axis, I need to set the lowest and the highest value limit which in this case are 0 and 100.
I’m working with percentages in this tutorial, but there are utility functions for data types other than numbers which I will explain later.
I have to split the height of the chart between these two values into equal parts. For this, I create something that is called a scaling function.
const yScale = d3.scaleLinear() .range([height, 0]) .domain([0, 100]);
Linear scale is the most commonly known scaling type. It converts a continuous input domain into a continuous output range. Notice the range
and domain
method. The first one takes the length that should be divided between the limits of the domain values.
Remember, the SVG coordinate system starts from the top left corner that’s why the range takes the height as the first parameter and not zero.
Creating an axis on the left is as simple as adding another group and calling d3’s axisLeft
method with the scaling function as a parameter.
chart.append('g') .call(d3.axisLeft(yScale));
Now, continue with the x-axis.
const xScale = d3.scaleBand() .range([0, width]) .domain(sample.map((s) => s.language)) .padding(0.2) chart.append('g') .attr('transform', `translate(0, ${height})`) .call(d3.axisBottom(xScale));
Be aware that I use scaleBand for the x-axis which helps to split the range into bands and compute the coordinates and widths of the bars with additional padding.
D3.js is also capable of handling date type among many others. scaleTime is really similar to scaleLinear except the domain is here an array of dates.
Drawing Bars in D3.js
Think about what kind of input we need to draw the bars. They each represent a value which is illustrated with simple shapes, specifically rectangles. In the next code snippet, I append them to the created group element.
chart.selectAll() .data(goals) .enter() .append('rect') .attr('x', (s) => xScale(s.language)) .attr('y', (s) => yScale(s.value)) .attr('height', (s) => height - yScale(s.value)) .attr('width', xScale.bandwidth())
First, I selectAll
elements on the chart which returns with an empty result set. Then, data
function tells how many elements the DOM should be updated with based on the array length. enter
identifies elements that are missing if the data input is longer than the selection. This returns a new selection representing the elements that need to be added. Usually, this is followed by an append
which adds elements to the DOM.
Basically, I tell D3.js to append a rectangle for every member of the array.
Now, this only adds rectangles on top of each other which have no height or width. These two attributes have to be calculated and that’s where the scaling functions come handy again.
See, I add the coordinates of the rectangles with the attr
call. The second parameter can be a callback which takes 3 parameters: the actual member of the input data, index of it and the whole input.
.attr(’x’, (actual, index, array) => xScale(actual.value))
The scaling function returns the coordinate for a given domain value. Calculating the coordinates are a piece of cake, the trick is with the height of the bar. The computed y coordinate has to be subtracted from the height of the chart to get the correct representation of the value as a column.
I define the width of the rectangles with the scaling function as well. scaleBand
has a bandwidth
function which returns the computed width for one element based on the set padding.
Nice job, but not so fancy, right?
To prevent our audience from eye bleeding, let’s add some info and improve the visuals! ;)
Tips on making bar charts
There are some ground rules with bar charts that worth mentioning.
- Avoid using 3D effects;
- Order data points intuitively - alphabetically or sorted;
- Keep distance between the bands;
- Start y-axis at 0 and not with the lowest value;
- Use consistent colors;
- Add axis labels, title, source line.
D3.js Grid System
I want to highlight the values by adding grid lines in the background.
Go ahead, experiment with both vertical and horizontal lines but my advice is to display only one of them. Excessive lines can be distracting. This code snippet presents how to add both solutions.
chart.append('g') .attr('class', 'grid') .attr('transform', `translate(0, ${height})`) .call(d3.axisBottom() .scale(xScale) .tickSize(-height, 0, 0) .tickFormat('')) chart.append('g') .attr('class', 'grid') .call(d3.axisLeft() .scale(yScale) .tickSize(-width, 0, 0) .tickFormat(''))
I prefer the vertical grid lines in this case because they lead the eyes and keep the overall picture plain and simple.
Labels in D3.js
I also want to make the diagram more comprehensive by adding some textual guidance. Let’s give a name to the chart and add labels for the axes.
Texts are SVG elements that can be appended to the SVG or groups. They can be positioned with x and y coordinates while text alignment is done with the text-anchor
attribute. To add the label itself, just call text
method on the text element.
svg.append('text') .attr('x', -(height / 2) - margin) .attr('y', margin / 2.4) .attr('transform', 'rotate(-90)') .attr('text-anchor', 'middle') .text('Love meter (%)') svg.append('text') .attr('x', width / 2 + margin) .attr('y', 40) .attr('text-anchor', 'middle') .text('Most loved programming languages in 2018')
Interactivity with D3.js
We got quite an informative chart but still, there are possibilities to make it interactive too.
In the next code block I show You how to add event listeners to SVG elements.
svgElement .on('mouseenter', function (actual, i) { d3.select(this).attr(‘opacity’, 0.5) }) .on('mouseleave’, function (actual, i) { d3.select(this).attr(‘opacity’, 1) })
Note that I use function expression instead of an arrow function because I access the element via this
keyword.
I set the opacity of the selected SVG element to half of the original value on mouse hover and reset it when the cursor leaves the area.
You could also get the mouse coordinates with d3.mouse
. It returns an array with the x and y coordinate. This way, displaying a tooltip at the tip of the cursor would be no problem at all.
Creating eye-popping diagrams is not an easy art form.
One might require the wisdom of graphic designers, UX researchers and other mighty creatures. In the following example I’m going to show a few possibilities to boost Your chart!
I have very similar values displayed on the chart so to highlight the divergences among the bar values, I set up an event listener for the mouseenter
event. Every time the user hovers over a specific a column, a horizontal line is drawn on top of that bar. Furthermore, I also calculate the differences compared to the other bands and display it on the bars.
Pretty neat, huh? I also added the opacity example to this one and increased the width of the bar.
.on(‘mouseenter’, function (s, i) { d3.select(this) .transition() .duration(300) .attr('opacity', 0.6) .attr('x', (a) => xScale(a.language) - 5) .attr('width', xScale.bandwidth() + 10) chart.append('line') .attr('x1', 0) .attr('y1', y) .attr('x2', width) .attr('y2', y) .attr('stroke', 'red') // this is only part of the implementation, check the source code })
The transition
method indicates that I want to animate changes to the DOM. Its interval is set with the duration
function that takes milliseconds as arguments. This transition above fades the band color and broaden the width of the bar.
To draw an SVG line, I need a start and a destination point. This can be set via the x1
, y1
and x2
, y2
coordinates. The line will not be visible until I set the color of it with the stroke
attribute.
I only revealed part of the mouseenter
event here so keep in mind, You have to revert or remove the changes on the mouseout
event. The full source code is available at the end of the article.
Let’s Add Some Style to the Chart!
Let’s see what we achieved so far and how can we shake up this chart with some style. You can add class attributes to SVG elements with the same attr
function we used before.
The diagram has a nice set of functionality. Instead of a dull, static picture, it also reveals the divergences among the represented values on mouse hover. The title puts the chart into context and the labels help to identify the axes with the unit of measurement. I also add a new label to the bottom right corner to mark the input source.
The only thing left is to upgrade the colors and fonts!
Charts with dark background makes the bright colored bars look cool. I also applied the Open Sans
font family to all the texts and set size and weight for the different labels.
Do You notice the line got dashed? It can be done by setting the stroke-width
and stroke-dasharray
attributes. With stroke-dasharray
, You can define pattern of dashes and gaps that alter the outline of the shape.
line#limit { stroke: #FED966; stroke-width: 3; stroke-dasharray: 3 6; } .grid path { stroke-width: 3; } .grid .tick line { stroke: #9FAAAE; stroke-opacity: 0.2; }
Grid lines where it gets tricky. I have to apply stroke-width: 0
to path elements in the group to hide the frame of the diagram and I also reduce their visibility by setting the opacity of the lines.
All the other css rules cover the font sizes and colors which You can find in the source code.
Wrapping up our D3.js Bar Chart Tutorial
D3.js is an amazing library for DOM manipulation. The depth of it hides countless hidden (actually not hidden, it is really well documented) treasures that waits for discovery. This writing covers only fragments of its toolset that help to create a not so mediocre bar chart.
Go on, explore it, use it and create spectacular visualizations!
By the way, here's the link to the source code.
Have You created something cool with D3.js? Share with us! Drop a comment if You have any questions or would like another tutorial on the topic!
Thanks for reading and see You next time!
:bar_chart: Hello Friends! :chart_with_upwards_trend:
We just released a new #javascript tutorial where we teach you how to use #d3js to create beautiful, interactive bar charts like this one :point_down::point_down: https://t.co/7uvYqWBKuk pic.twitter.com/OofIyelyYV
— RisingStack :beers: Node.js, K8S & Microservices (@RisingStack) August 1, 2018Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK