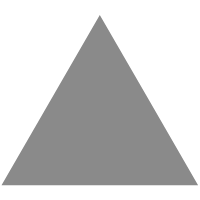
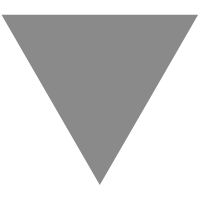
Difference Between BeanFactory and ApplicationContext in Spring
source link: https://www.tuicool.com/articles/hit/IrYjInJ
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
I see a lot of questions asking about the difference between BeanFactory and ApplicationContext.
Along with that, I get the question: should I use the former or the latter to get beans from the Spring container?
We previously talked about the Spring container here . Basically, these two interfaces supply the way to reach Spring beans from the container, but there are some significant differences.
Let's take a look!
What Is a Spring Bean?
This is a very simple question that is often overcomplicated. Usually, Spring beans are Java objects that are managed by the Spring container.
Here is a simple Spring bean example:
package com.zoltanraffai; public class HelloWorld { private String message; public void setMessage(String message){ this.message = message; } public void getMessage(){ System.out.println("My Message : " + message); } }
In the XML-based configuration, beans.xml supplies the metadata for the Spring container to manage the bean.
What Is the Spring Container?
The Spring container is responsible for instantiating, configuring, and assembling the Spring beans. Here is an example of how we configure our HelloWorld POJO for the IoC container:
<?xml version = "1.0" encoding = "UTF-8"?> <beans xmlns = "http://www.springframework.org/schema/beans" xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation = "http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd"> <bean id = "helloWorld" class = "com.zoltanraffai.HelloWorld"> <property name = "message" value = "Hello World!"/> </bean> </beans>
Now, it managed by the Spring container. The only question is: how we can access it?
The Difference Between BeanFactory and ApplicationContext
The BeanFactory Interface
This is the root interface for accessing the Spring container. To access the Spring container, we will be using Spring's dependency injection functionality using this BeanFactory interface and its sub-interfaces.
Features:
- Bean instantiation/wiring
It is important to mention that the BeanFactory interface only supports XML-based bean configuration. Usually, the implementations use lazy loading, which means that beans are only instantiating when we directly calling them through the getBean()
method.
The most used API that implements the BeanFactory is the XmlBeanFactory .
Here is an example of how to get a bean through the BeanFactory:
package com.zoltanraffai; import org.springframework.core.io.ClassPathResource; import org.springframework.beans.factory.InitializingBean; import org.springframework.beans.factory.xml.XmlBeanFactory; public class HelloWorldApp{ public static void main(String[] args) { XmlBeanFactory factory = new XmlBeanFactory (new ClassPathResource("beans.xml")); HelloWorld obj = (HelloWorld) factory.getBean("helloWorld"); obj.getMessage(); } }
The ApplicationContext Interface
The ApplicationContext is the central interface within a Spring application that is used for providing configuration information to the application.
It implements the BeanFactory interface. Hence, the ApplicationContext includes all functionality of the BeanFactory and much more! Its main function is to support the creation of big business applications.
Features:
- Bean instantiation/wiring
- Automatic BeanPostProcessor registration
- Automatic BeanFactoryPostProcessor registration
- Convenient MessageSource access (for i18n)
- ApplicationEvent publication
The ApplicationContext supports both XML and annotation-based bean configuration. It
uses eager loading, so every bean instantiate after the ApplicationContext is started up.
Here is an example of the ApplicationContext usage:
package com.zoltanraffai; import org.springframework.core.io.ClassPathResource; import org.springframework.beans.factory.InitializingBean; import org.springframework.beans.factory.xml.XmlBeanFactory; public class HelloWorldApp{ public static void main(String[] args) { ApplicationContext context=new ClassPathXmlApplicationContext("beans.xml"); HelloWorld obj = (HelloWorld) String.getBean("helloWorld"); obj.getMessage(); } }
Conclusion
The ApplicationContext includes all the functionality of the BeanFactory. It is generally recommended to use the former. There are some limited situations, such as in mobile applications, where memory consumption might be critical. In those scenarios, it would be justifiable to use the more lightweight BeanFactory . However, in most enterprise applications, the ApplicationContext is what you will want to use.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK