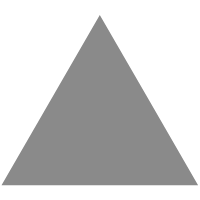
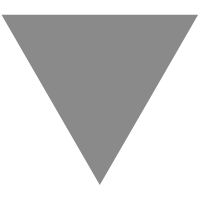
Laravel Query Builder
source link: https://www.tuicool.com/articles/hit/YFriIrU
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Laravel Query Builder is a package by Alex Vanderbist and the folks at Spatie, to quickly build Eloquent queries from API requests. This package makes creating complex API queries with Laravel incredibly simple. While you may have already heard of this package (or used it), I wanted to make sure everyone is aware of this one!
I highly recommend you take Query Builder for a spin and read through the README to learn what it’s capable of doing. It makes creating API filters and other query-related tasks so easy, you might feel like you’re cheating.
A few basic examples of what you can do include filtering an API request, including related models, incorporating it with existing queries, sorting an API request.
For example, using the request /users?filter[name]=John
, here’s how you can use filtering on the name
field in the users
table:
use Spatie\QueryBuilder\QueryBuilder; // ... $users = QueryBuilder::for(User::class) ->allowedFilters('name') ->get(); // all `User`s that contain the string "John" in their name
If you have an existing query, you can pass it along to the query builder:
$query = User::where('active', true); $user = QueryBuilder::for($query) ->allowedIncludes('posts', 'permissions') ->where('score', '>', 42) // chain on any of Laravel's query builder methods ->first();
A more advanced example and one of my favorite features in this package are the scope filters. Say that you have the following scope:
public function scopeStartsBefore(Builder $query, $date): Builder { return $query->where('starts_at', '>=', Carbon::parse($date)); }
The associated query builder might look something like this:
QueryBuilder::for(Event::class) ->allowedFilters([ Filter::scope('starts_before'), ]) ->get();
With the above query builder, it’s now possible to make the following query to filter results on the starts_before
column like so:
GET /events?filter[starts_before]=2018-01-01
Learn More
You can view the source code and read the documentation on how to install and use this package on GitHub at spatie/laravel-query-builder . The examples found in this article are from the Laravel Query Builder README file, and I’d encourage you to read through the whole thing for more details.
You can install this package in your Laravel 5 project with the following composer command:
composer require spatie/laravel-query-builder
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK