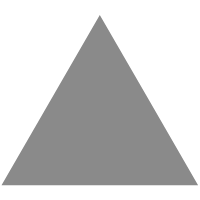
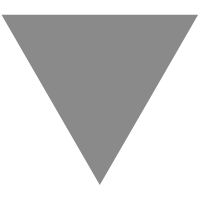
React's New Component Lifecycle
source link: https://www.tuicool.com/articles/hit/MbUzeaq
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Not long agoAnkit Kumar wrotean excellent article about React's component lifecycle. One of the things about technology is that IT changes, and changes quickly. React's current stable version is 16.4.1 , and with it came some significant changes planned and implemented for the lifecycle hooks .
I am somewhat new to React and have been building a project in which I decided to use the new lifecycle hooks. This article discusses the new changes, and my experience switching from the old lifecycle hooks to the new lifecycle hooks as well as a pitfall that I hope to help you avoid.
The following hooks will be deprecated, and ultimately removed: componentWillMount
, componentWillReceiveProps
, componentWillUpdate
Before you panic, know that it will be a long time before these hooks are completely deprecated and removed from React. Your current use of the component hooks will work as expected even if you update your React version to the latest stable release.
So the question is: with these hooks ultimately going away what hooks will be replacing them? This is where the pitfall I spoke of earlier comes in. The replacement lifecycle hooks
are not a one-to-one replacement
. There are two new lifecycle hooks: getDerivedStateFromProps
and getSnapshotBeforeUpdate
My initial understanding was that getDerivedStateFromProps
is a direct replacement for componentWillReceiveProps
. The way that React's blog is written makes it seem that way:
getDerivedStateFromProps
is being added as a safer alternative to the legacy componentWillReceiveProps
.
There is a React blog post that came
after
this that explained that you probably don't need derived state
. That knowledge would have been a nice to have before I changed all my componentWillRecieveProps
to the shiny new replacement getDerivedStateFromProps
.
The reason the three lifecycle hooks are being deprecated is because the React team realized that in many cases they were being used incorrectly by developers in the first place. With these changes we now have six component hooks:
-
componentDidMount(): void
-
static getDerivedStateFromProps(object nextProps, object prevState): null | object
-
componentDidUpdate(object prevProps, object prevState, mixed snapShot): void
-
componentWillUnmount(): void
-
getSnapshotBeforeUpdate(object prevProps, object prevState): mixed
-
render(): JSX | false
Let's go over each of these hooks in some detail:
componentDidMount(): void
This hook is unchanged, and fires when the component has loaded into the DOM. You can make changes to DOM elements here. Also, to change state you need to use setState()
.
static getDerivedStateFromProps(object nextProps, object prevState):
null | object
Intended as a safe replacement for componentWillReceiveProps()
-- however, this is not meant as a one-to-one replacement. Many times the sole use of componentWillReceiveProps
is to set state based on the incoming property changes. If that's only
what your original hook did then getDerivedStateFromProps
may
be a good replacement.
The first thing to notice about this new hook is that it is static. What this means is that you are prevented from calling methods and accessing properties in the class. If the state should change then you should return a state object; which is like calling this.setState({stateName: value})
If the state does not need to change then you should return a null. In my projects the only time I use getDerivedStateFromProps
is in a modal class:
/** * Lifecycle hook getDerivedStateFromProps * * @param {object} nextProps * @return {object | null} */ static getDerivedStateFromProps(nextProps) { if (nextProps.memberInfo && nextProps.show) { return {memberInfo: {...nextProps.memberInfo}, shouldShow: true}; } return null; }
The code above will update state only if the incoming properties memberInfo
and show
are truthy. This makes sense for a modal component, since the only time it should change state from external property changes is if it is showing, and, in my case, if the memberInfo
object is ready to be edited in the modal form.
componentDidUpdate(object prevProps, object prevState, mixed snapShot): void
A third argument has been added to this component hook: snapShot
. This third argument (if it exists) will be the return value from the new getSnapshotBeforeUpdate
lifecycle hook.
You can update state in this hook via setState()
, and this hook can
be used in conjunction with getDerivedStateFromProps
that sets state from the props, and then use componentDidUpdate
to check for changes in state such as the record Id change.
componentWillUnmount(): void
No changes were made to this component hook. You can not change state here, and this is basically a deconstructor event hook for React components where you would do any clean up needed before the component exists and is cleared out of memory.
getSnapshotBeforeUpdate(object prevProps, object prevState): mixed
This new component hook
is "added to support safely reading properties from the DOM before updates are made." The example given in the React blog is where you want to keep and restore the scroll position in your component as it refreshes. The way it does this is the return value can be any valid JavaScript value. The return value then becomes the third argument in the componentDidUpdate
hook. I as of yet have not needed to use this new component hook.
render(): JSX | false
The change to render
is that it can now return false. Previously, the only valid return value for render
is JSX. Now, if you return false in the render
component hook it will not render anything. This makes for cleaner conditional rendering code.
Hopefully, this short intro to the new component lifecycle hooks has been helpful. Check out React's blog for more details and examples.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK