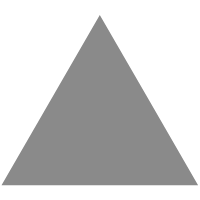
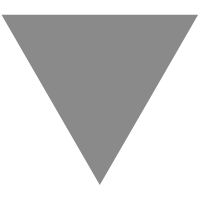
The Easiest Way To Add Validation To Your Forms
source link: https://www.tuicool.com/articles/hit/26Rn6zR
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Adding validation to your HTML forms will make sure your users enter their data accurately and in the correct format. In the past, validating form inputs required the use of JavaScript plugins, but today most browsers have built-in solutions that can handle most of the validation.
In this tutorial we will show you how to add validation rules to your forms, using only native HTML input attributes.
Project Overview
To demonstrate the process of creating validation rules, we have prepared a simple HTML registration form using Bootstrap 4 . You can click the image below to open a live demo.
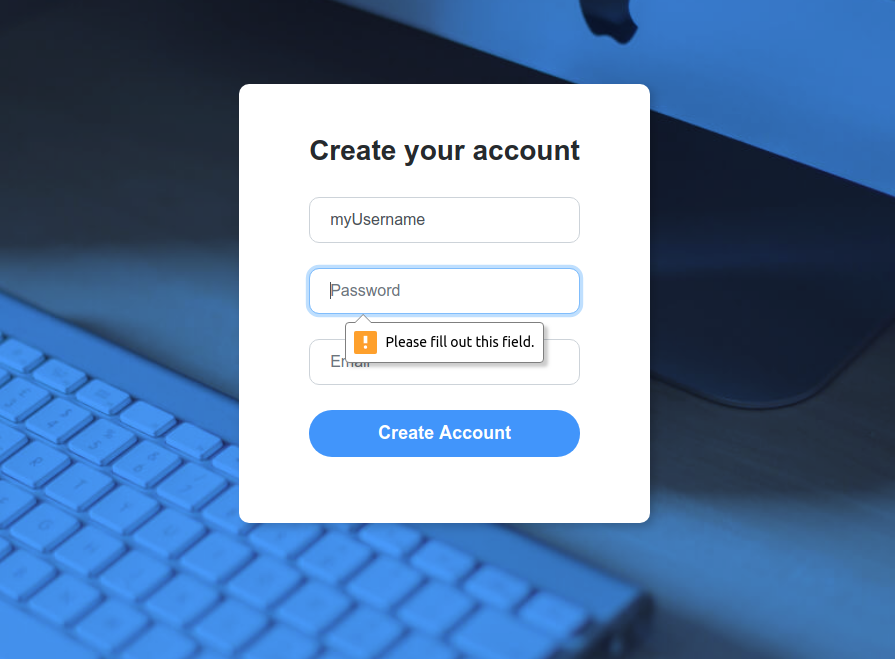
Layout
HTML
Our design consists of a Bootstrap form with a couple of input fields and a submit button. For this example we have used 3 different types of input fields - a text input, a password input, and an email input.
<div class="registration-form"> <form> <h3 class="text-center">Create your account</h3> <div class="form-group"> <input class="form-control item" type="text" name="username" maxlength="15" minlength="4" pattern="^[a-zA-Z0-9_.-]*$" id="username" placeholder="Username" required> </div> <div class="form-group"> <input class="form-control item" type="password" name="password" minlength="6" id="password" placeholder="Password" required> </div> <div class="form-group"> <input class="form-control item" type="email" name="email" id="email" placeholder="Email" required> </div> <div class="form-group"> <button class="btn btn-primary btn-block create-account" type="submit">Create Account</button> </div> </form> </div>
CSS
We've also added some basic CSS styles to make the form a bit easier on the eyes.
html { background-color:#214c84; background-blend-mode:overlay; display:flex; align-items:center; justify-content:center; background-image:url(../../assets/img/image4.jpg); background-repeat:no-repeat; background-size:cover; height:100%; } body { background-color:transparent; } .registration-form { padding:50px 0; } .registration-form form { max-width:800px; padding:50px 70px; border-radius:10px; box-shadow:4px 4px 15px rgba(0, 0, 0, 0.2); background-color:#fff; } .registration-form form h3 { font-weight:bold; margin-bottom:30px; } .registration-form .item { border-radius:10px; margin-bottom:25px; padding:10px 20px; } .registration-form .create-account { border-radius:30px; padding:10px 20px; font-size:18px; font-weight:bold; background-color:#3f93ff; border:none; color:white; margin-top:20px; } @media (max-width: 576px) { .registration-form form { padding:50px 20px; } }
Validation Types
HTML5 offers a nice and easy way for inline validation, using input attributes. There is a large number of attributes available, we will only take a look at some of the most common. For a full list you can visit this excellent MDN guide .
required
This attribute specifies that the input field can not be empty. It requires the user to enter something before submitting the form.
<input class="form-control item" type="email" name="email" id="email" placeholder="Email" required>
maxlength and minlength
Specifies the maximum/minimum number of symbols that the user can enter in the input field. This is especially useful in password fields where a longer password means a safer password.
<input class="form-control item" type="password" name="password" minlength="6" id="password" placeholder="Password" required>
pattern
Specifies a regular expression that has to be matched in order for the entered data to pass. It can be used with the following input types: text, search, url, email, and password. If you are not familiar with regular expressions you can check out our tutorial Learn Regular Expressions in 20 Minutes .
<input class="form-control item" type="text" name="username" maxlength="15" minlength="4" pattern="^[a-zA-Z0-9_.-]*$" id="username" placeholder="Username" required>
Form Validation in Bootstrap Studio
Bootstrap Studio offers a fast and easy way to validate your forms without having to write a single line of code. The app has built-in controls that allow you to quickly setup all the validation rules you may need.
For a more detailed tutorial on how to create form validation in your projects head out to the Bootstrap Studio website.
Conclusion
You can get the full source code for this tutorial, from the Download button near the top of the page. You are free to customize and use it in all your projects, for commercial or personal use, no attribution required (our license).
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK