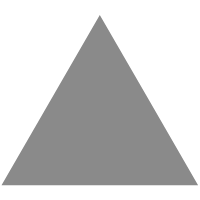
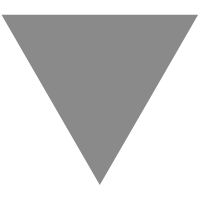
Getting Started With the Fabric Python Library
source link: https://www.tuicool.com/articles/hit/N7Bfuuz
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Fabric is a Python library and command-line tool for streamlining the use of SSH for application deployment or systems administration tasks. Fabric is very simple and powerful and can help to automate repetitive command-line tasks. This approach can save time by automating your entire workflow.
This tutorial will cover how to use Fabric to integrate with SSH and automate tasks.
Installation
Fabric is best installed via pip:
$ pip install fabric
Getting Started With Fabric
Usage
Below is a simple function demonstrating how to use Fabric.
def welcome(): print("Welcome to getting started with Fabric!")
The program above is then saved as fabfile.py
in your current working directory. The welcome function can be executed with the fab
tool as follows:
$ fab welcome Welcome to getting started with Fabric
Fabric provides the fab command which reads its configuration from a file, fabfile.py
. The file should be in the directory from which the command is run. A standard fabfile contains the functions to be executed on a remote host or a group of remote hosts.
Features
Fabric implements functions which can be used to communicate with remote hosts:
fabric.operations.run()
This operation is used to run a shell command on a remote host.
Examples
run("ls /var/www/") run("ls /home/userx", shell=False) output = run('ls /var/www/mysites'
fabric.operations.get()
This function is used to download file(s) from a remote host. The example below shows how to download a backup from a remote server.
# Downloading a back-up get("/backup/db.bak", "./db.bak")
fabric.operations.put()
This functions uploads file(s) to a remote host. For example:
with cd('/tmp'): put('/path/to/local/test.txt', 'files')
fabric.operations.reboot()
As the name suggests, this function reboots a system server.
# Reboot the remote system reboot()
fabric.operations.sudo()
This function is used to execute commands on a remote host with superuser privileges. Additionally, you can also pass an additional user argument which allows you to run commands as another user other than root.
Example
# Create a directory sudo("mkdir /var/www")
fabric.operations.local()
This function is used to run a command on the local system. An example is:
# Extract the contents of a tar archive local("tar xzvf /tmp/trunk/app.tar.gz") # Remove a file local("rm /tmp/trunk/app.tar.gz")
fabric.operations.prompt()
The function prompts the user with text and returns the input.
Examples
# Simplest form: environment = prompt('Please specify target environment: ') # specify host env_host = prompt('Please specify host:')
fabric.operations.require()
This function is used to check for given keys in a shared environment dict. If not found, the operation is aborted.
SSH Integration
One of the ways developers interact with remote servers besides FTP clients is through SSH. SSH is used to connect to remote servers and do everything from basic configuration to running Git or initiating a web server.
With Fabric, you can perform SSH activities from your local computer.
The example below defines functions that show how to check free disk space and host type. It also defines which host will run the command:
# Import Fabric's API module from fabric.api import run env.hosts = '159.89.39.54' # Set the username env.user = "root" def host_type(): run('uname -s') def diskspace(): run('df') def check(): # check host type host_type() # Check diskspace diskspace()
In order to run this code, you will need to run the following command on the terminal:
fab check
fab check[159.89.39.54] Executing task 'check' [159.89.39.54] run: uname -s [159.89.39.54] Login password for 'root': [159.89.39.54] out: Linux [159.89.39.54] out: [159.89.39.54] run: df [159.89.39.54] out: Filesystem 1K-blocks Used Available Use% Mounted on [159.89.39.54] out: udev 242936 0 242936 0% /dev [159.89.39.54] out: tmpfs 50004 6020 43984 13% /run [159.89.39.54] out: /dev/vda1 20145768 4398716 15730668 22% / [159.89.39.54] out: tmpfs 250012 1004 249008 1% /dev/shm [159.89.39.54] out: tmpfs 5120 0 5120 0% /run/lock [159.89.39.54] out: tmpfs 250012 0 250012 0% /sys/fs/cgroup [159.89.39.54] out: /dev/vda15 106858 3426 103433 4% /boot/efi [159.89.39.54] out: tmpfs 50004 0 50004 0% /run/user/0 [159.89.39.54] out: none 20145768 4398716 15730668 22% /var/lib/docker/aufs/mnt/781d1ce30963c0fa8af93b5679bf96425a0a10039d10be8e745e1a22a9909105 [159.89.39.54] out: shm 65536 0 65536 0% /var/lib/docker/containers/036b6bcd5344f13fdb1fc738752a0850219c7364b1a3386182fead0dd8b7460b/shm [159.89.39.54] out: none 20145768 4398716 15730668 22% /var/lib/docker/aufs/mnt/17934c0fe3ba83e54291c1aebb267a2762ce9de9f70303a65b12f808444dee80 [159.89.39.54] out: shm 65536 0 65536 0% /var/lib/docker/containers/fd90146ad4bcc0407fced5e5fbcede5cdd3cff3e96ae951a88f0779ec9c2e42d/shm [159.89.39.54] out: none 20145768 4398716 15730668 22% /var/lib/docker/aufs/mnt/ba628f525b9f959664980a73d94826907b7df31d54c69554992b3758f4ea2473 [159.89.39.54] out: shm 65536 0 65536 0% /var/lib/docker/containers/dbf34128cafb1a1ee975f56eb7637b1da0bfd3648e64973e8187ec1838e0ea44/shm [159.89.39.54] out: Done. Disconnecting from 159.89.39.54... done.
Automating Tasks
Fabric enables you to run commands on a remote server without needing to log in to the remote server.
Remote execution with Fabric can lead to security threats since it requires an open SSH port, especially on Linux machines.
For instance, let's assume you want to update the system libraries on your remote server. You don't necessarily need to execute the tasks every other time. You can just write a simple fab file which you will run every time you want to execute the tasks.
In this case, you will first import the Fabric API's module:
from fabric.api import *
Define the remote host you want to update:
env.hosts = '159.89.39.54'
Set the username of the remote host:
env.user = "root"
Although it's not recommended, you might need to specify the password to the remote host.
Lastly, define the function that updates the libraries in your remote host.
def update_libs(): """ Update the default OS installation's basic default tools. """ run("apt-get update")
Now that your fab file is ready, all you need to do is execute it as follows:
$ fab update
You should see the following result:
$ fab update [159.89.39.54] Executing task 'update' [159.89.39.54] run: apt-get update [159.89.39.54] Login password for 'root':
If you didn't define the password, you will be prompted for it.
After the program has finished executing the defined commands, you will get the following response, if no errors occur:
$ fab update ............ Disconnecting from 159.89.39.54... done.
Conclusion
This tutorial has covered what is necessary to get started with Fabric locally and on remote hosts. You can now confidently start writing your own scripts for building, monitoring or maintaining remote servers.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK