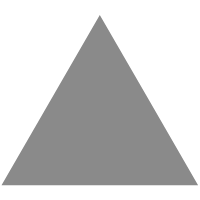
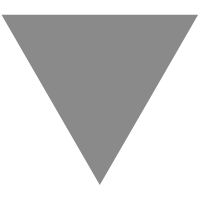
GitHub - ganderzz/react-scroll-to: Scroll to a position in React
source link: https://github.com/ganderzz/react-scroll-to
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
React Scroll-To
A React component that makes scrolling easy.
React Scroll-To provides a Higher Order Component, and Render Props implementation.
Example: View React Storybook Examples
Install
npm: npm i react-scroll-to --save
yarn: yarn add react-scroll-to
Render Props:
// Scroll to position (20, 500) in the browser window
import React, { Component } from "react";
import { ScrollTo } from "react-scroll-to";
export default class MyComponent extends Component {
render() {
return (
<ScrollTo>
{({ scroll }) => (
<a onClick={() => scroll({ x: 20, y: 500 })}>Scroll to Bottom</a>
)}
</ScrollTo>
);
}
}
// Scroll to position (0, 500) within all provided <ScrollArea /> children
import React, { Component } from "react";
import { ScrollTo, ScrollArea } from "react-scroll-to";
export default class MyComponent extends Component {
render() {
return (
<ScrollTo>
{({ scroll }) => (
<ScrollArea style={{ height: 1000 }}>
<button onClick={() => scroll({ y: 500, smooth: true })}>
Scroll within this container
</button>
</ScrollArea>
)}
</ScrollTo>
);
}
}
// Scroll to position (0, 500) within a specific <ScrollArea /> child
import React, { Component } from "react";
import { ScrollTo, ScrollArea } from "react-scroll-to";
export default class MyComponent extends Component {
render() {
return (
<ScrollTo>
{({ scroll }) => (
<div>
<ScrollArea id="foo" style={{ height: 1000 }}>
<button onClick={() => scroll({ id: "foo", y: 500 })}>
Scroll within this container
</button>
</ScrollArea>
<ScrollArea style={{ height: 1000 }}>
This container won't scroll
</ScrollArea>
</div>
)}
</ScrollTo>
);
}
}
// Scroll by a component's ref
import React, { Component } from "react";
import { ScrollTo } from "react-scroll-to";
export default class MyComponent extends Component {
myRef = React.createRef();
render() {
return (
<>
<ScrollTo>
{({ scroll }) => (
<a onClick={() => scroll({ ref: this.myRef, x: 20, y: 500 })}>
Scroll to Bottom
</a>
)}
</ScrollTo>
<div ref={this.myRef}>My Element</div>
</>
);
}
}
Higher Order Component:
// Scroll to position (0, 500) within the browser window
import React from "react";
import { ScrollToHOC } from "react-scroll-to";
export default ScrollToHOC(function(props) {
return <a onClick={() => props.scroll({ y: 500 })}>Scroll to Bottom</a>;
});
// Scroll to position (0, 500) within all provided <ScrollArea /> children
import React from "react";
import { ScrollToHOC, ScrollArea } from "react-scroll-to";
export default ScrollToHOC(function(props) {
return (
<ScrollArea style={{ height: 1000 }}>
<a onClick={() => props.scroll({ y: 500 })}>Scroll to Bottom</a>
</ScrollArea>
);
});
// Scroll to position (0, 500) within a specific <ScrollArea /> child
import React from "react";
import { ScrollToHOC, ScrollArea } from "react-scroll-to";
export default ScrollToHOC(function(props) {
return (
<div>
<ScrollArea id="foo" style={{ height: 1000 }}>
<a onClick={() => props.scroll({ id: "foo", y: 500 })}>
Scroll to Bottom
</a>
</ScrollArea>
<ScrollArea style={{ height: 1000 }}>
This container won't scroll
</ScrollArea>
</div>
);
});
Types:
- Typescript definitions are built in
- Flow is currently not support (Open for PRs!)
2.0 Changes
- v2.0 has a new API for controlling scrolling. Instead of taking two arguments, x and y, the ScrollTo component now takes an object.
scrollTo({
x: 25 // The horizontal x position to scroll to
y: 10 // The vertical y position to scroll to
id: "myId" // The ID of the ScrollArea we want to scroll
ref: refObj // A reference to a component to scroll
smooth: true // If true, this will animate the scroll to be smooth. False will give an instant scroll. (defaults: false)
})
Mixing and matching these options give different results.
- The
scrollById
function has been deprecated in favor of theid
field inscrollTo
Smooth Scrolling Not Working?
Some browsers don't natively support smooth scroll. Checkout adding a polyfill like smoothscroll-polyfill
to fix the issue.
npm install smoothscroll-polyfill
Contributors
Thanks goes to these wonderful people (emoji key):
This project follows the all-contributors specification. Contributions of any kind welcome!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK