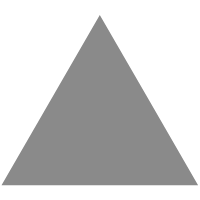
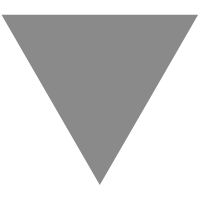
GitHub - JedS6391/LGP: A robust LGP implementation on the JVM using Kotlin.
source link: https://github.com/JedS6391/LGP
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
A robust LGP implementation on the JVM using Kotlin.
About
An implementation of Linear Genetic Programming that follows that outlined by Linear Genetic Programming (Brameier, M. F. and Banzhaf, W. 2007).
The framework is implemented in Kotlin which allows for easily interoperability with Java (and other JVM languages), while adding the benefit of modern programming language features.
To get started with how to use the framework, see the documentation.
If you find the framework useful or have any queries, please feel free to:
Installation
Note: The LGP framework requires JDK 8 (Java 1.8).
A JAR containing the core API can be downloaded from the releases page. Each version will have its artefact uploaded here.
Alternatively, the package is available on Maven central, so you can reference the package as a dependency using the format appropriate for your package manager (see here for a full list). For example, to add to an existing Gradle build script:
repositories {
mavenCentral()
}
dependencies {
// Core abstractions and components of the LGP framework
compile "nz.co.jedsimson.lgp:core:<VERSION>"
// To get the full source, include the sources package
compile "nz.co.jedsimson.lgp:core:<VERSION>:sources"
// Implementations for core LGP framework components
compile "nz.co.jedsimson.lgp:lib:<VERSION>"
// To get the full source, include the sources package
compile "nz.co.jedsimson.lgp:lib:<VERSION>:sources"
}
Tests
The test suite for the framework can be run with the following gradle command:
./gradlew test --info --rerun-tasks
Usage
Examples
A set of example usages can be found in the LGP-examples repository. The examples cover a few different problem configurations, including:
- Programs with a single or multiple outputs
- Reading dataset from a file
- Generating a dataset
- Custom fitness functions
- Usage from Java
Getting started
The framework is built using Kotlin and the easiest way to use it is through the Kotlin API. Instructions for installation and usage of the Kotlin compiler, kotlinc
, can be found for the Command Line or IntelliJ IDEA.
Here, we'll focus on how to use the framework through Kotlin (particularly from the command line) but documentation is provided for using the API through Java. This guide assumes you want to directly use the JAR file and not through another build system.
Assuming that kotlinc
is installed and available at the command line, the first step is to download the core API JAR file as described in the Installation section. You will also want to download the LGP-lib package which provides implementations of core components, particularly BaseProblem
which we will use in this example.
Next, create a blank Kotlin file that will contain the problem definition --- typically this would have a filename matching that of the problem:
touch MyProblem.kt
We're not going to fully define the problem as that would be a needlessly extensive exercise, so we'll simply show how to import classes from the API and build against the imported classes.
In MyProblem.kt
, enter the following content:
import nz.co.jedsimson.lgp.core.environment.config.Configuration
import nz.co.jedsimson.lgp.core.evolution.Description
import nz.co.jedsimson.lgp.lib.base.BaseProblem
import nz.co.jedsimson.lgp.lib.base.BaseProblemParameters
fun main(args: Array<String>) {
val parameters = BaseProblemParameters(
name = "My Problem",
description = Description("A simple example problem definition"),
config = Configuration()
)
val problem = BaseProblem(parameters)
println(problem.name)
println(problem.description)
}
Here, we use the BaseProblem
implementation to use a default set of parameters that we can quickly test against using a data set (which is omitted here).
To compile, we use kotlinc
:
kotlinc -cp LGP-core.jar:LGP-lib.jar -no-jdk -no-stdlib MyProblem.kt
This will generate a class file in the directory called MyProblemKt.class
. To interpret the class file using the Kotlin interpreter is simple:
kotlin -cp LGP-core.jar:LGP-lib.jar:. MyProblemKt
You should see the following output:
My Problem
Description(description=A simple example problem definition)
Please refer to the usage guide for instructions on using the API from the context of a Java program.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK